GitHub - eslint/eslint: Find and fix problems in your JavaScript code.Find and fix problems in your JavaScript code. Contribute to eslint/eslint development by creating an account on GitHub.
Visit Site
GitHub - eslint/eslint: Find and fix problems in your JavaScript code.
ESLint
Website | Configure ESLint | Rules | Contribute to ESLint | Report Bugs | Code of Conduct | Twitter | Discord | Mastodon
ESLint is a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code. In many ways, it is similar to JSLint and JSHint with a few exceptions:
- ESLint uses Espree for JavaScript parsing.
- ESLint uses an AST to evaluate patterns in code.
- ESLint is completely pluggable, every single rule is a plugin and you can add more at runtime.
Table of Contents
- Installation and Usage
- Configuration
- Version Support
- Code of Conduct
- Filing Issues
- Frequently Asked Questions
- Releases
- Security Policy
- Semantic Versioning Policy
- Stylistic Rule Updates
- License
- Team
- Sponsors
- Technology Sponsors
Installation and Usage
Prerequisites: Node.js (^18.18.0
, ^20.9.0
, or >=21.1.0
) built with SSL support. (If you are using an official Node.js distribution, SSL is always built in.)
You can install and configure ESLint using this command:
npm init @eslint/config@latest
After that, you can run ESLint on any file or directory like this:
npx eslint yourfile.js
Configuration
You can configure rules in your eslint.config.js
files as in this example:
export default [
{
files: ["**/*.js", "**/*.cjs", "**/*.mjs"],
rules: {
"prefer-const": "warn",
"no-constant-binary-expression": "error"
}
}
];
The names "prefer-const"
and "no-constant-binary-expression"
are the names of rules in ESLint. The first value is the error level of the rule and can be one of these values:
"off"
or0
- turn the rule off"warn"
or1
- turn the rule on as a warning (doesn't affect exit code)"error"
or2
- turn the rule on as an error (exit code will be 1)
The three error levels allow you fine-grained control over how ESLint applies rules (for more configuration options and details, see the configuration docs).
Version Support
The ESLint team provides ongoing support for the current version and six months of limited support for the previous version. Limited support includes critical bug fixes, security issues, and compatibility issues only.
ESLint offers commercial support for both current and previous versions through our partners, Tidelift and HeroDevs.
See Version Support for more details.
Code of Conduct
ESLint adheres to the OpenJS Foundation Code of Conduct.
Filing Issues
Before filing an issue, please be sure to read the guidelines for what you're reporting:
Frequently Asked Questions
Does ESLint support JSX?
Yes, ESLint natively supports parsing JSX syntax (this must be enabled in configuration). Please note that supporting JSX syntax is not the same as supporting React. React applies specific semantics to JSX syntax that ESLint doesn't recognize. We recommend using eslint-plugin-react if you are using React and want React semantics.
Does Prettier replace ESLint?
No, ESLint and Prettier have different jobs: ESLint is a linter (looking for problematic patterns) and Prettier is a code formatter. Using both tools is common, refer to Prettier's documentation to learn how to configure them to work well with each other.
What ECMAScript versions does ESLint support?
ESLint has full support for ECMAScript 3, 5, and every year from 2015 up until the most recent stage 4 specification (the default). You can set your desired ECMAScript syntax and other settings (like global variables) through configuration.
What about experimental features?
ESLint's parser only officially supports the latest final ECMAScript standard. We will make changes to core rules in order to avoid crashes on stage 3 ECMAScript syntax proposals (as long as they are implemented using the correct experimental ESTree syntax). We may make changes to core rules to better work with language extensions (such as JSX, Flow, and TypeScript) on a case-by-case basis.
In other cases (including if rules need to warn on more or fewer cases due to new syntax, rather than just not crashing), we recommend you use other parsers and/or rule plugins. If you are using Babel, you can use @babel/eslint-parser and @babel/eslint-plugin to use any option available in Babel.
Once a language feature has been adopted into the ECMAScript standard (stage 4 according to the TC39 process), we will accept issues and pull requests related to the new feature, subject to our contributing guidelines. Until then, please use the appropriate parser and plugin(s) for your experimental feature.
Which Node.js versions does ESLint support?
ESLint updates the supported Node.js versions with each major release of ESLint. At that time, ESLint's supported Node.js versions are updated to be:
- The most recent maintenance release of Node.js
- The lowest minor version of the Node.js LTS release that includes the features the ESLint team wants to use.
- The Node.js Current release
ESLint is also expected to work with Node.js versions released after the Node.js Current release.
Refer to the Quick Start Guide for the officially supported Node.js versions for a given ESLint release.
Where to ask for help?
Open a discussion or stop by our Discord server.
Why doesn't ESLint lock dependency versions?
Lock files like package-lock.json
are helpful for deployed applications. They ensure that dependencies are consistent between environments and across deployments.
Packages like eslint
that get published to the npm registry do not include lock files. npm install eslint
as a user will respect version constraints in ESLint's package.json
. ESLint and its dependencies will be included in the user's lock file if one exists, but ESLint's own lock file would not be used.
We intentionally don't lock dependency versions so that we have the latest compatible dependency versions in development and CI that our users get when installing ESLint in a project.
The Twilio blog has a deeper dive to learn more.
Releases
We have scheduled releases every two weeks on Friday or Saturday. You can follow a release issue for updates about the scheduling of any particular release.
Security Policy
ESLint takes security seriously. We work hard to ensure that ESLint is safe for everyone and that security issues are addressed quickly and responsibly. Read the full security policy.
Semantic Versioning Policy
ESLint follows semantic versioning. However, due to the nature of ESLint as a code quality tool, it's not always clear when a minor or major version bump occurs. To help clarify this for everyone, we've defined the following semantic versioning policy for ESLint:
- Patch release (intended to not break your lint build)
- A bug fix in a rule that results in ESLint reporting fewer linting errors.
- A bug fix to the CLI or core (including formatters).
- Improvements to documentation.
- Non-user-facing changes such as refactoring code, adding, deleting, or modifying tests, and increasing test coverage.
- Re-releasing after a failed release (i.e., publishing a release that doesn't work for anyone).
- Minor release (might break your lint build)
- A bug fix in a rule that results in ESLint reporting more linting errors.
- A new rule is created.
- A new option to an existing rule that does not result in ESLint reporting more linting errors by default.
- A new addition to an existing rule to support a newly-added language feature (within the last 12 months) that will result in ESLint reporting more linting errors by default.
- An existing rule is deprecated.
- A new CLI capability is created.
- New capabilities to the public API are added (new classes, new methods, new arguments to existing methods, etc.).
- A new formatter is created.
eslint:recommended
is updated and will result in strictly fewer linting errors (e.g., rule removals).
- Major release (likely to break your lint build)
eslint:recommended
is updated and may result in new linting errors (e.g., rule additions, most rule option updates).- A new option to an existing rule that results in ESLint reporting more linting errors by default.
- An existing formatter is removed.
- Part of the public API is removed or changed in an incompatible way. The public API includes:
- Rule schemas
- Configuration schema
- Command-line options
- Node.js API
- Rule, formatter, parser, plugin APIs
According to our policy, any minor update may report more linting errors than the previous release (ex: from a bug fix). As such, we recommend using the tilde (~
) in package.json
e.g. "eslint": "~3.1.0"
to guarantee the results of your builds.
Stylistic Rule Updates
Stylistic rules are frozen according to our policy on how we evaluate new rules and rule changes. This means:
- Bug fixes: We will still fix bugs in stylistic rules.
- New ECMAScript features: We will also make sure stylistic rules are compatible with new ECMAScript features.
- New options: We will not add any new options to stylistic rules unless an option is the only way to fix a bug or support a newly-added ECMAScript feature.
License
Team
These folks keep the project moving and are resources for help.
Technical Steering Committee (TSC)
The people who manage releases, review feature requests, and meet regularly to ensure ESLint is properly maintained.
Reviewers
The people who review and implement new features.
Committers
The people who review and fix bugs and help triage issues.
Website Team
Team members who focus specifically on eslint.org
Sponsors
The following companies, organizations, and individuals support ESLint's ongoing maintenance and development. Become a Sponsor to get your logo on our READMEs and website.
More Resourcesto explore the angular.
mail [email protected] to add your project or resources here 🔥.
- 1[unmaintained] DalekJS Base framework
https://github.com/dalekjs/dalek
[unmaintained] DalekJS Base framework. Contribute to dalekjs/dalek development by creating an account on GitHub.
- 2Tabulator - Interactive JavaScript Tables
http://olifolkerd.github.io/tabulator/
Create interactive data tables in seconds with Tabulator. A lightweight, fully featured JQuery table generation plugin.
- 3A simple and stable cross-browser testing tool. 简单稳定的跨浏览器测试工具。
https://github.com/totorojs/totoro
A simple and stable cross-browser testing tool. 简单稳定的跨浏览器测试工具。 - totorojs/totoro
- 4A next-generation package manager for the front-end
https://github.com/duojs/duo
A next-generation package manager for the front-end - duojs/duo
- 5The HTML Presentation Framework
https://github.com/hakimel/reveal.js
The HTML Presentation Framework. Contribute to hakimel/reveal.js development by creating an account on GitHub.
- 6PixiJS | The HTML5 Creation Engine | PixiJS
https://pixijs.com
PixiJS - The HTML5 Creation Engine. Create beautiful digital content with the fastest, most flexible 2D WebGL renderer.
- 7AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more.
https://github.com/adonisjs/core
AdonisJS is a TypeScript-first web framework for building web apps and API servers. It comes with support for testing, modern tooling, an ecosystem of official packages, and more. - adonisjs/core
- 8OS.js - JavaScript Web Desktop Platform
https://github.com/os-js/OS.js
OS.js - JavaScript Web Desktop Platform. Contribute to os-js/OS.js development by creating an account on GitHub.
- 9A rugged, minimal framework for composing JavaScript behavior in your markup.
https://github.com/alpinejs/alpine
A rugged, minimal framework for composing JavaScript behavior in your markup. - GitHub - alpinejs/alpine: A rugged, minimal framework for composing JavaScript behavior in your markup.
- 10Phaser - A fast, fun and free open source HTML5 game framework
https://phaser.io
Desktop and Mobile HTML5 game framework. A fast, free and fun open source framework for Canvas and WebGL powered browser games.
- 11BDD / TDD assertion framework for node.js and the browser that can be paired with any testing framework.
https://github.com/chaijs/chai
BDD / TDD assertion framework for node.js and the browser that can be paired with any testing framework. - chaijs/chai
- 12Polymaps is a free JavaScript library for making dynamic, interactive maps in modern web browsers.
https://github.com/simplegeo/polymaps
Polymaps is a free JavaScript library for making dynamic, interactive maps in modern web browsers. - simplegeo/polymaps
- 13List.js
https://listjs.com
Perfect library for adding search, sort, filters and flexibility to tables, lists and various HTML elements. Built to be invisible and work on existing HTML.
- 14Javascript panorama viewer based on Three.js
https://github.com/pchen66/panolens.js
Javascript panorama viewer based on Three.js. Contribute to pchen66/panolens.js development by creating an account on GitHub.
- 15JavaScript Library for creating random pleasing colors and color schemes
https://github.com/Fooidge/PleaseJS
JavaScript Library for creating random pleasing colors and color schemes - Fooidge/PleaseJS
- 16Build CRUD apps in fewer lines of code.
https://github.com/canjs/canjs
Build CRUD apps in fewer lines of code. Contribute to canjs/canjs development by creating an account on GitHub.
- 17File Upload widget with multiple file selection, drag&drop support, progress bar, validation and preview images, audio and video for jQuery. Supports cross-domain, chunked and resumable file uploads. Works with any server-side platform (Google App Engine, PHP, Python, Ruby on Rails, Java, etc.) that supports standard HTML form file uploads.
https://github.com/blueimp/jQuery-File-Upload
File Upload widget with multiple file selection, drag&drop support, progress bar, validation and preview images, audio and video for jQuery. Supports cross-domain, chunked and resumable file up...
- 18Create an Apple-like one page scroller website (iPhone 5S website) with One Page Scroll plugin
https://github.com/peachananr/onepage-scroll
Create an Apple-like one page scroller website (iPhone 5S website) with One Page Scroll plugin - peachananr/onepage-scroll
- 19FoalTS
https://foalts.org
Full-featured Node.js framework, with no complexity
- 20A frontend framework for chillout-mode development 🥤 JSX components on generators*, fast mobx-like state management and exclusive cssx style system
https://github.com/whatsup/whatsup
A frontend framework for chillout-mode development 🥤 JSX components on generators*, fast mobx-like state management and exclusive cssx style system - whatsup/whatsup
- 21JavaScript library for all kinds of color manipulations
https://github.com/gka/chroma.js
JavaScript library for all kinds of color manipulations - gka/chroma.js
- 22Matter.js
https://brm.io/matter-js
Matter.js is 2D rigid body physics engine for the web, using JavaScript and HTML5
- 23Validate your forms, frontend, without writing a single line of javascript
https://github.com/guillaumepotier/Parsley.js
Validate your forms, frontend, without writing a single line of javascript - guillaumepotier/Parsley.js
- 24PEG.js: Parser generator for JavaScript
https://github.com/pegjs/pegjs
PEG.js: Parser generator for JavaScript. Contribute to pegjs/pegjs development by creating an account on GitHub.
- 25qTip2 - Pretty powerful tooltips
https://github.com/qTip2/qTip2
qTip2 - Pretty powerful tooltips. Contribute to qTip2/qTip2 development by creating an account on GitHub.
- 26Integrated end-to-end testing framework written in Node.js and using W3C Webdriver API. Developed at @browserstack
https://github.com/nightwatchjs/nightwatch
Integrated end-to-end testing framework written in Node.js and using W3C Webdriver API. Developed at @browserstack - nightwatchjs/nightwatch
- 27Simple HTML5 Charts using the <canvas> tag
https://github.com/chartjs/Chart.js
Simple HTML5 Charts using the <canvas> tag. Contribute to chartjs/Chart.js development by creating an account on GitHub.
- 28Scriptable Headless Browser
https://github.com/ariya/phantomjs
Scriptable Headless Browser. Contribute to ariya/phantomjs development by creating an account on GitHub.
- 29The superpowered headless CMS for Node.js — built with GraphQL and React
https://github.com/keystonejs/keystone
The superpowered headless CMS for Node.js — built with GraphQL and React - keystonejs/keystone
- 30AnyChart is a lightweight and robust JavaScript charting library
https://www.anychart.com/
AnyChart is a lightweight and robust JavaScript charting solution with great API and documentation. The chart types and unique features are numerous, and the library works easily with any development stack.
- 31Independent technology for modern publishing, memberships, subscriptions and newsletters.
https://github.com/tryghost/Ghost
Independent technology for modern publishing, memberships, subscriptions and newsletters. - TryGhost/Ghost
- 32A modern dialog library which is highly configurable and easy to style. #hubspot-open-source
https://github.com/HubSpot/vex
A modern dialog library which is highly configurable and easy to style. #hubspot-open-source - HubSpot/vex
- 33A-Frame – Make WebVR
https://aframe.io
A web framework for building virtual reality experiences. Make WebVR with HTML and Entity-Component. Works on Vive, Rift, desktop, mobile platforms.
- 34The Backbone Framework
https://github.com/marionettejs/backbone.marionette
The Backbone Framework. Contribute to marionettejs/backbone.marionette development by creating an account on GitHub.
- 35[Deprecated] See @go-catupiri as a direct golang port
https://github.com/wejs/we/
[Deprecated] See @go-catupiri as a direct golang port - wejs/we
- 36Magic number detection for JavaScript
https://github.com/danielstjules/buddy.js
Magic number detection for JavaScript. Contribute to danielstjules/buddy.js development by creating an account on GitHub.
- 37Home page | Yarn
https://yarnpkg.com/
Yarn, the modern JavaScript package manager
- 38Create front end projects from templates, add dependencies, and automate the resulting projects
https://github.com/volojs/volo
Create front end projects from templates, add dependencies, and automate the resulting projects - volojs/volo
- 39A visualization grammar. Moved to: https://github.com/vega/vega
https://github.com/trifacta/vega
A visualization grammar. Moved to: https://github.com/vega/vega - trifacta/vega
- 40Chance - Random generator helper for JavaScript
https://github.com/chancejs/chancejs
Chance - Random generator helper for JavaScript. Contribute to chancejs/chancejs development by creating an account on GitHub.
- 41Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API.
https://github.com/microsoft/playwright
Playwright is a framework for Web Testing and Automation. It allows testing Chromium, Firefox and WebKit with a single API. - GitHub - microsoft/playwright: Playwright is a framework for Web Testi...
- 42Optimize React performance and make your React 70% faster in minutes, not months.
https://github.com/aidenybai/million
Optimize React performance and make your React 70% faster in minutes, not months. - GitHub - aidenybai/million: Optimize React performance and make your React 70% faster in minutes, not months.
- 43Simple flow library 🖥️🖱️
https://github.com/jerosoler/Drawflow
Simple flow library 🖥️🖱️. Contribute to jerosoler/Drawflow development by creating an account on GitHub.
- 44Fast, disk space efficient package manager | pnpm
https://pnpm.io/
Fast, disk space efficient package manager
- 45Modular JavaScript Utilities
https://github.com/mout/mout
Modular JavaScript Utilities. Contribute to mout/mout development by creating an account on GitHub.
- 46Tiny vanilla JS plugin to display large data sets easily
https://github.com/NeXTs/Clusterize.js
Tiny vanilla JS plugin to display large data sets easily - NeXTs/Clusterize.js
- 47Snowpack
https://www.snowpack.dev/
Snowpack is a lightning-fast frontend build tool, designed for the modern web.
- 48A modern JavaScript utility library delivering modularity, performance, & extras.
https://github.com/lodash/lodash
A modern JavaScript utility library delivering modularity, performance, & extras. - lodash/lodash
- 49bundlejs
https://bundle.js.org
Visit bundlejs.com - bundlejs is a quick and easy way to bundle, minify, and compress your ts, js, and npm packages all online.
- 50The API and real-time application framework
https://github.com/feathersjs/feathers
The API and real-time application framework. Contribute to feathersjs/feathers development by creating an account on GitHub.
- 51The same DOM API and Frameworks you know, but in a Web Worker.
https://github.com/ampproject/worker-dom
The same DOM API and Frameworks you know, but in a Web Worker. - ampproject/worker-dom
- 52Dynamic ES module loader
https://github.com/systemjs/systemjs
Dynamic ES module loader. Contribute to systemjs/systemjs development by creating an account on GitHub.
- 53DEPRECATED: Timezone-enabled JavaScript Date object. Uses Olson zoneinfo files for timezone data.
https://github.com/mde/timezone-js
DEPRECATED: Timezone-enabled JavaScript Date object. Uses Olson zoneinfo files for timezone data. - mde/timezone-js
- 54HTML5 application architecture using Backbone.js
https://github.com/chaplinjs/chaplin
HTML5 application architecture using Backbone.js. Contribute to chaplinjs/chaplin development by creating an account on GitHub.
- 55Lightweight, robust, elegant syntax highlighting.
https://github.com/PrismJS/prism
Lightweight, robust, elegant syntax highlighting. Contribute to PrismJS/prism development by creating an account on GitHub.
- 56WebdriverIO · Next-gen browser and mobile automation test framework for Node.js | WebdriverIO
https://webdriver.io/
Next-gen browser and mobile automation test framework for Node.js
- 57Console.log with style.
https://github.com/adamschwartz/log
Console.log with style. Contribute to adamschwartz/log development by creating an account on GitHub.
- 58Lightweight and easy to use the library for modals
https://github.com/Alexandrshy/keukenhof
Lightweight and easy to use the library for modals - Alexandrshy/keukenhof
- 59JSHint is a tool that helps to detect errors and potential problems in your JavaScript code
https://github.com/jshint/jshint/
JSHint is a tool that helps to detect errors and potential problems in your JavaScript code - jshint/jshint
- 60ml5 - A friendly machine learning library for the web.
https://ml5js.org
ml5.js aims to make machine learning approachable for a broad audience of artists, creative coders, and students. The library provides access to machine learning algorithms and models in the browser, building on top of TensorFlow.js with no other external dependencies.
- 61A responsive image polyfill for <picture>, srcset, sizes, and more
https://github.com/scottjehl/picturefill
A responsive image polyfill for <picture>, srcset, sizes, and more - scottjehl/picturefill
- 62A general purpose, real-time visualization library.
https://github.com/epochjs/epoch
A general purpose, real-time visualization library. - epochjs/epoch
- 63ES Module Package Manager
https://github.com/jspm/jspm-cli
ES Module Package Manager. Contribute to jspm/jspm-cli development by creating an account on GitHub.
- 64⛔️ DEPRECATED - Dependency-free notification library that makes it easy to create alert - success - error - warning - information - confirmation messages as an alternative the standard alert dialog.
https://github.com/needim/noty
⛔️ DEPRECATED - Dependency-free notification library that makes it easy to create alert - success - error - warning - information - confirmation messages as an alternative the standard alert dialog...
- 65E2E test framework for Angular apps
https://github.com/angular/protractor
E2E test framework for Angular apps. Contribute to angular/protractor development by creating an account on GitHub.
- 66PlayCanvas - The Web Graphics Creation Platform
https://playcanvas.com
Collaboratively build stunning HTML5 visualizations and games
- 67DevDocs
https://devdocs.io/
Fast, offline, and free documentation browser for developers. Search 100+ docs in one web app including HTML, CSS, JavaScript, PHP, Ruby, Python, Go, C, C++, and many more.
- 68A modern approach for Computer Vision on the web
https://github.com/eduardolundgren/tracking.js
A modern approach for Computer Vision on the web. Contribute to eduardolundgren/tracking.js development by creating an account on GitHub.
- 69:fork_and_knife: Web applications made easy. Since 2011.
https://github.com/brunch/brunch
:fork_and_knife: Web applications made easy. Since 2011. - brunch/brunch
- 70A tiny javascript templating framework in ~400 bytes gzipped
https://github.com/jasonmoo/t.js
A tiny javascript templating framework in ~400 bytes gzipped - jasonmoo/t.js
- 71Simple, lightweight, persistent two-way databinding
https://github.com/gwendall/way.js
Simple, lightweight, persistent two-way databinding - gwendall/way.js
- 72jQuery feature tour plugin.
https://github.com/zurb/joyride
jQuery feature tour plugin. Contribute to zurb/joyride development by creating an account on GitHub.
- 732012 UI framework (I was 20 years old, React didn't exist, inspired by Knockout)
https://github.com/astoilkov/jsblocks
2012 UI framework (I was 20 years old, React didn't exist, inspired by Knockout) - astoilkov/jsblocks
- 74Lightweight JavaScript form validation library inspired by CodeIgniter.
https://github.com/rickharrison/validate.js
Lightweight JavaScript form validation library inspired by CodeIgniter. - rickharrison/validate.js
- 75Lightweight and powerful data binding.
https://github.com/mikeric/rivets
Lightweight and powerful data binding. Contribute to mikeric/rivets development by creating an account on GitHub.
- 76Magically convert a simple text input into a cool tag list with this jQuery plugin.
https://github.com/xoxco/jQuery-Tags-Input
Magically convert a simple text input into a cool tag list with this jQuery plugin. - xoxco/jQuery-Tags-Input
- 77Simple JavaScript testing framework for browsers and node.js
https://github.com/jasmine/jasmine
Simple JavaScript testing framework for browsers and node.js - jasmine/jasmine
- 78JavaScript Charts & Maps - amCharts
https://www.amcharts.com/
JavaScript charts and maps data-viz libraries for web, dashboards, and applications. Fast and flexible. React/Angular compatible. Since 2006.
- 79Lightweight, vanilla javascript parallax library
https://github.com/dixonandmoe/rellax
Lightweight, vanilla javascript parallax library. Contribute to dixonandmoe/rellax development by creating an account on GitHub.
- 80Port of FFmpeg with Emscripten
https://github.com/Kagami/ffmpeg.js
Port of FFmpeg with Emscripten. Contribute to Kagami/ffmpeg.js development by creating an account on GitHub.
- 81Create word clouds in JavaScript.
https://github.com/jasondavies/d3-cloud
Create word clouds in JavaScript. Contribute to jasondavies/d3-cloud development by creating an account on GitHub.
- 82Learn, Build, & Test RegEx
https://regexr.com/
RegExr is an online tool to learn, build, & test Regular Expressions (RegEx / RegExp).
- 83The missing Javascript smart persistent layer
https://github.com/Wisembly/basil.js
The missing Javascript smart persistent layer. Contribute to Wisembly/basil.js development by creating an account on GitHub.
- 84Pre-evaluate code at build-time with babel-macros
https://github.com/kentcdodds/preval.macro
Pre-evaluate code at build-time with babel-macros. Contribute to kentcdodds/preval.macro development by creating an account on GitHub.
- 85The zero configuration build tool for the web. 📦🚀
https://github.com/parcel-bundler/parcel
The zero configuration build tool for the web. 📦🚀. Contribute to parcel-bundler/parcel development by creating an account on GitHub.
- 86Embedded CoffeeScript templates
https://github.com/sstephenson/eco/
Embedded CoffeeScript templates. Contribute to sstephenson/eco development by creating an account on GitHub.
- 87JSCover is a JavaScript Code Coverage Tool that measures line, branch and function coverage
https://github.com/tntim96/JSCover
JSCover is a JavaScript Code Coverage Tool that measures line, branch and function coverage - tntim96/JSCover
- 88:arrow_heading_up: JavaScript Code Style checker (unmaintained)
https://github.com/jscs-dev/node-jscs
:arrow_heading_up: JavaScript Code Style checker (unmaintained) - jscs-dev/node-jscs
- 89:dizzy: TransitionEnd is an agnostic and cross-browser library to work with transitionend event.
https://github.com/EvandroLG/transitionEnd
:dizzy: TransitionEnd is an agnostic and cross-browser library to work with transitionend event. - EvandroLG/transitionEnd
- 90Very lightweight progress bars. No jQuery
https://github.com/jacoborus/nanobar
Very lightweight progress bars. No jQuery. Contribute to jacoborus/nanobar development by creating an account on GitHub.
- 91🔥 JavaScript Library for HTML5 canvas based heatmaps
https://github.com/pa7/heatmap.js
🔥 JavaScript Library for HTML5 canvas based heatmaps - pa7/heatmap.js
- 92Minimalistic BDD-style assertions for Node.JS and the browser.
https://github.com/Automattic/expect.js
Minimalistic BDD-style assertions for Node.JS and the browser. - Automattic/expect.js
- 93The only script in your HEAD.
https://github.com/headjs/headjs
The only script in your HEAD. Contribute to headjs/headjs development by creating an account on GitHub.
- 94enterprise standard loader
https://github.com/ecomfe/esl
enterprise standard loader. Contribute to ecomfe/esl development by creating an account on GitHub.
- 95Attractive JavaScript charts for jQuery
https://github.com/flot/flot
Attractive JavaScript charts for jQuery. Contribute to flot/flot development by creating an account on GitHub.
- 96Prettier is an opinionated code formatter.
https://github.com/prettier/prettier
Prettier is an opinionated code formatter. Contribute to prettier/prettier development by creating an account on GitHub.
- 97:skull: An ancient tiny JS and CSS loader from the days before everyone had written one. Unmaintained.
https://github.com/rgrove/lazyload/
:skull: An ancient tiny JS and CSS loader from the days before everyone had written one. Unmaintained. - rgrove/lazyload
- 98Asynchronous Javascript templating for the browser and server
https://github.com/linkedin/dustjs/
Asynchronous Javascript templating for the browser and server - linkedin/dustjs
- 99Popline is an HTML5 Rich-Text-Editor Toolbar
https://github.com/kenshin54/popline
Popline is an HTML5 Rich-Text-Editor Toolbar. Contribute to kenshin54/popline development by creating an account on GitHub.
- 100A collection of awesome derby components
https://github.com/russll/awesome-derby
A collection of awesome derby components. Contribute to russll/awesome-derby development by creating an account on GitHub.
- 101The Type Linter for JS
https://github.com/getify/TypL
The Type Linter for JS. Contribute to getify/TypL development by creating an account on GitHub.
- 102Git hooks made easy 🐶 woof!
https://github.com/typicode/husky
Git hooks made easy 🐶 woof! Contribute to typicode/husky development by creating an account on GitHub.
- 103The perfect library for adding search, sort, filters and flexibility to tables, lists and various HTML elements. Built to be invisible and work on existing HTML.
https://github.com/javve/list.js
The perfect library for adding search, sort, filters and flexibility to tables, lists and various HTML elements. Built to be invisible and work on existing HTML. - javve/list.js
- 104For formatting, searching, and rewriting JavaScript.
https://github.com/rdio/jsfmt
For formatting, searching, and rewriting JavaScript. - rdio/jsfmt
- 105A tiny foundation for building reactive views
https://github.com/ripplejs/ripple
A tiny foundation for building reactive views. Contribute to ripplejs/ripple development by creating an account on GitHub.
- 106:new_moon: Lune.js — Calculate the phases of the moon
https://github.com/ryanseys/lune
:new_moon: Lune.js — Calculate the phases of the moon - ryanseys/lune
- 107AutoAnimate - Add motion to your apps with a single line of code
https://auto-animate.formkit.com
A zero-config, drop-in animation utility that automatically adds smooth transitions to your web app. Use it with React, Solid, Vue, Svelte, or any other JavaScript application.
- 108MVC framework making it easy to write realtime, collaborative applications that run in both Node.js and browsers
https://github.com/derbyjs/derby
MVC framework making it easy to write realtime, collaborative applications that run in both Node.js and browsers - derbyjs/derby
- 109A lightweight, easy-to-use jQuery plugin for fluid width video embeds.
https://github.com/davatron5000/FitVids.js
A lightweight, easy-to-use jQuery plugin for fluid width video embeds. - davatron5000/FitVids.js
- 110anime.js
https://animejs.com/
Javascript animation engine
- 111functional/point-free utils for JavaScript
https://github.com/alanrsoares/prelude-js
functional/point-free utils for JavaScript. Contribute to alanrsoares/prelude-js development by creating an account on GitHub.
- 112JavaScript framework for visual programming
https://github.com/retejs/rete
JavaScript framework for visual programming. Contribute to retejs/rete development by creating an account on GitHub.
- 113:flashlight: Set a spotlight focus on DOM element adding a overlay layer to the rest of the page
https://github.com/zzarcon/focusable
:flashlight: Set a spotlight focus on DOM element adding a overlay layer to the rest of the page - zzarcon/focusable
- 114frontend package manager and build tool for modular web applications
https://github.com/componentjs/component
frontend package manager and build tool for modular web applications - componentjs/component
- 115clipboard.js
https://clipboardjs.com/
A modern approach to copy text to clipboard. No Flash. No frameworks. Just 3kb gzipped
- 116A Module Loader for the Web
https://github.com/seajs/seajs
A Module Loader for the Web. Contribute to seajs/seajs development by creating an account on GitHub.
- 117A scriptable browser like PhantomJS, based on Firefox
https://github.com/laurentj/slimerjs
A scriptable browser like PhantomJS, based on Firefox - laurentj/slimerjs
- 118📦 Zero-configuration bundler for tiny modules.
https://github.com/developit/microbundle
📦 Zero-configuration bundler for tiny modules. Contribute to developit/microbundle development by creating an account on GitHub.
- 119Multi-Dimensional charting built to work natively with crossfilter rendered with d3.js
https://github.com/dc-js/dc.js
Multi-Dimensional charting built to work natively with crossfilter rendered with d3.js - dc-js/dc.js
- 120Test spies, stubs and mocks for JavaScript.
https://github.com/sinonjs/sinon
Test spies, stubs and mocks for JavaScript. Contribute to sinonjs/sinon development by creating an account on GitHub.
- 121CSS3 list scroll effects
https://github.com/hakimel/stroll.js
CSS3 list scroll effects. Contribute to hakimel/stroll.js development by creating an account on GitHub.
- 122A CSS only tooltip library for your lovely websites.
https://github.com/chinchang/hint.css
A CSS only tooltip library for your lovely websites. - chinchang/hint.css
- 123Cybernetically enhanced web apps
https://github.com/sveltejs/svelte
Cybernetically enhanced web apps. Contribute to sveltejs/svelte development by creating an account on GitHub.
- 124Asyncronous JavaScript loader and dependency manager
https://github.com/ded/script.js
Asyncronous JavaScript loader and dependency manager - ded/script.js
- 125TaracotJS Instance Generator
https://github.com/xtremespb/taracotjs-generator/
TaracotJS Instance Generator. Contribute to xtremespb/taracotjs-generator development by creating an account on GitHub.
- 126Ember.js - A JavaScript framework for creating ambitious web applications
https://github.com/emberjs/ember.js
Ember.js - A JavaScript framework for creating ambitious web applications - emberjs/ember.js
- 127Design your web audio player, the way you want.
https://521dimensions.com/open-source/amplitudejs
AmplitudeJS empowers designers to have full control of the web audio experience. Free on Github.
- 128Parallax Engine that reacts to the orientation of a smart device
https://github.com/wagerfield/parallax
Parallax Engine that reacts to the orientation of a smart device - wagerfield/parallax
- 129JavaScript模块加载器,基于AMD。迄今为止,对AMD理解最好的实现。
https://github.com/yanhaijing/lodjs
JavaScript模块加载器,基于AMD。迄今为止,对AMD理解最好的实现。. Contribute to yanhaijing/lodjs development by creating an account on GitHub.
- 130Give your JS App some Backbone with Models, Views, Collections, and Events
https://github.com/jashkenas/backbone
Give your JS App some Backbone with Models, Views, Collections, and Events - jashkenas/backbone
- 131A build system for development of composable software.
https://github.com/teambit/bit
A build system for development of composable software. - teambit/bit
- 132O JavaScript que você deveria conhecer.
https://medium.com/@pedropolisenso/o-javasscript-que-voc%C3%AA-deveria-conhecer-b70e94d1d706
Não ser tão técnico te faz ser um diferencial no mercado, acredita nisso?
- 133noUiSlider is a lightweight, ARIA-accessible JavaScript range slider with multi-touch and keyboard support. It is fully GPU animated: no reflows, so it is fast; even on older devices. It also fits wonderfully in responsive designs and has no dependencies.
https://github.com/leongersen/noUiSlider
noUiSlider is a lightweight, ARIA-accessible JavaScript range slider with multi-touch and keyboard support. It is fully GPU animated: no reflows, so it is fast; even on older devices. It also fits ...
- 134A markdown editor. http://lab.lepture.com/editor/
https://github.com/lepture/editor
A markdown editor. http://lab.lepture.com/editor/. Contribute to lepture/editor development by creating an account on GitHub.
- 135Node.js test runner that lets you develop with confidence 🚀
https://github.com/avajs/ava
Node.js test runner that lets you develop with confidence 🚀 - avajs/ava
- 136Find and fix problems in your JavaScript code.
https://github.com/eslint/eslint
Find and fix problems in your JavaScript code. Contribute to eslint/eslint development by creating an account on GitHub.
- 137ECMAScript parsing infrastructure for multipurpose analysis
https://github.com/ariya/esprima
ECMAScript parsing infrastructure for multipurpose analysis - ariya/esprima
- 138Tiny bootstrap-compatible WISWYG rich text editor
https://github.com/mindmup/bootstrap-wysiwyg
Tiny bootstrap-compatible WISWYG rich text editor. Contribute to mindmup/bootstrap-wysiwyg development by creating an account on GitHub.
- 139Simple JavaScript Duckumentation generator.
https://github.com/senchalabs/jsduck
Simple JavaScript Duckumentation generator. Contribute to senchalabs/jsduck development by creating an account on GitHub.
- 140Lo-fi, powerful, community-driven string manipulation library.
https://github.com/plexis-js/plexis
Lo-fi, powerful, community-driven string manipulation library. - plexis-js/plexis
- 141A javascript standard data structure library which benchmark against C++ STL.
https://github.com/zly201/js-sdsl
A javascript standard data structure library which benchmark against C++ STL. - ZLY201/js-sdsl
- 142A new interface to replace your old boring scrollbar
https://github.com/s-yadav/ScrollMenu
A new interface to replace your old boring scrollbar - s-yadav/ScrollMenu
- 143🗃 Offload your store management to a worker easily.
https://github.com/developit/stockroom
🗃 Offload your store management to a worker easily. - developit/stockroom
- 144▶️ Streams a list of tracks from Youtube, Soundcloud, Vimeo...
https://github.com/adrienjoly/playemjs
▶️ Streams a list of tracks from Youtube, Soundcloud, Vimeo... - adrienjoly/playemjs
- 145the no-library library: open module JavaScript framework
https://github.com/ender-js/Ender
the no-library library: open module JavaScript framework - ender-js/Ender
- 146AngularJS - HTML enhanced for web apps!
https://github.com/angular/angular.js
AngularJS - HTML enhanced for web apps! Contribute to angular/angular.js development by creating an account on GitHub.
- 147:ram: Practical functional Javascript
https://github.com/ramda/ramda
:ram: Practical functional Javascript. Contribute to ramda/ramda development by creating an account on GitHub.
- 148⚡ Empowering JavaScript with native platform APIs. ✨ Best of all worlds (TypeScript, Swift, Objective C, Kotlin, Java, Dart). Use what you love ❤️ Angular, Capacitor, Ionic, React, Solid, Svelte, Vue with: iOS (UIKit, SwiftUI), Android (View, Jetpack Compose), Dart (Flutter) and you name it compatible.
https://github.com/NativeScript/NativeScript
⚡ Empowering JavaScript with native platform APIs. ✨ Best of all worlds (TypeScript, Swift, Objective C, Kotlin, Java, Dart). Use what you love ❤️ Angular, Capacitor, Ionic, React, Solid, Svelte, V...
- 149:clock8: timeago.js is a tiny(2.0 kb) library used to format date with `*** time ago` statement.
https://github.com/hustcc/timeago.js
:clock8: :hourglass: timeago.js is a tiny(2.0 kb) library used to format date with `*** time ago` statement. - GitHub - hustcc/timeago.js: :clock8: timeago.js is a tiny(2.0 kb) library used to form...
- 150Next-gen browser and mobile automation test framework for Node.js
https://github.com/webdriverio/webdriverio
Next-gen browser and mobile automation test framework for Node.js - webdriverio/webdriverio
- 151Blazing fast Apple TV application development using pure JavaScript
https://github.com/emadalam/atvjs
Blazing fast Apple TV application development using pure JavaScript - emadalam/atvjs
- 152In-browser code editor (version 5, legacy)
https://github.com/codemirror/CodeMirror
In-browser code editor (version 5, legacy). Contribute to codemirror/codemirror5 development by creating an account on GitHub.
- 153JavaScript Client-Side Cookie Manipulation Library
https://github.com/ScottHamper/Cookies
JavaScript Client-Side Cookie Manipulation Library - ScottHamper/Cookies
- 154A lightweight and amazing WYSIWYG JavaScript editor under 10kB
https://github.com/Alex-D/Trumbowyg
A lightweight and amazing WYSIWYG JavaScript editor under 10kB - Alex-D/Trumbowyg
- 155Quill is a modern WYSIWYG editor built for compatibility and extensibility
https://github.com/quilljs/quill
Quill is a modern WYSIWYG editor built for compatibility and extensibility - slab/quill
- 156⏱ A library for working with dates and times in JS
https://github.com/moment/luxon
⏱ A library for working with dates and times in JS - moment/luxon
- 157:rainbow: Javascript color conversion and manipulation library
https://github.com/Qix-/color
:rainbow: Javascript color conversion and manipulation library - Qix-/color
- 158human friendly i18n for javascript (node.js + browser)
https://github.com/nodeca/babelfish/
human friendly i18n for javascript (node.js + browser) - nodeca/babelfish
- 159A simple CSS tooltip made with Sass
https://github.com/arashmanteghi/simptip
A simple CSS tooltip made with Sass. Contribute to arashmanteghi/simptip development by creating an account on GitHub.
- 160Insanely fast, full-stack, headless browser testing using node.js
https://github.com/assaf/zombie
Insanely fast, full-stack, headless browser testing using node.js - assaf/zombie
- 161Deprecated - Chosen is a library for making long, unwieldy select boxes more friendly.
https://github.com/harvesthq/chosen
Deprecated - Chosen is a library for making long, unwieldy select boxes more friendly. - harvesthq/chosen
- 162The worlds smallest fully-responsive css framework
https://github.com/mrmrs/fluidity
The worlds smallest fully-responsive css framework - mrmrs/fluidity
- 163:woman: Library for image processing
https://github.com/davidsonfellipe/lena.js
:woman: Library for image processing. Contribute to davidsonfellipe/lena.js development by creating an account on GitHub.
- 164ClojureScript's persistent data structures and supporting API from the comfort of vanilla JavaScript
https://github.com/swannodette/mori
ClojureScript's persistent data structures and supporting API from the comfort of vanilla JavaScript - swannodette/mori
- 165🍃 JavaScript library for mobile-friendly interactive maps 🇺🇦
https://github.com/Leaflet/Leaflet
🍃 JavaScript library for mobile-friendly interactive maps 🇺🇦 - Leaflet/Leaflet
- 166A simple and fast API to monitor elements as you scroll
https://github.com/stutrek/scrollMonitor
A simple and fast API to monitor elements as you scroll - stutrek/scrollmonitor
- 167A jQuery UI plugin to handle multi-tag fields as well as tag suggestions/autocomplete.
https://github.com/aehlke/tag-it
A jQuery UI plugin to handle multi-tag fields as well as tag suggestions/autocomplete. - aehlke/tag-it
- 168SurveyJS - JavaScript Libraries for Surveys and Forms
https://surveyjs.io/
Open-source JavaScript form builder libraries that allows you to create unlimited survey forms in a self-hosted form management system with a no-code drag-and-drop interface.
- 169A file and module loader for JavaScript
https://github.com/requirejs/requirejs
A file and module loader for JavaScript. Contribute to requirejs/requirejs development by creating an account on GitHub.
- 170Javascript Content Management System running on Node.js
https://github.com/jcoppieters/cody
Javascript Content Management System running on Node.js - jcoppieters/cody
- 171Super simple countdowns.
https://github.com/gumroad/countdown.js
Super simple countdowns. Contribute to gumroad/countdown.js development by creating an account on GitHub.
- 172Tiny & beautiful site-wide progress indicator
https://github.com/buunguyen/topbar
Tiny & beautiful site-wide progress indicator. Contribute to buunguyen/topbar development by creating an account on GitHub.
- 173A lightweight clientside JSON document store,
https://github.com/brianleroux/lawnchair/
A lightweight clientside JSON document store,. Contribute to brianleroux/lawnchair development by creating an account on GitHub.
- 174The Missing Javascript Datatable for the Web
https://github.com/frappe/datatable
The Missing Javascript Datatable for the Web. Contribute to frappe/datatable development by creating an account on GitHub.
- 175Vest ✅ Declarative validations framework
https://github.com/ealush/vest
Vest ✅ Declarative validations framework. Contribute to ealush/vest development by creating an account on GitHub.
- 176Learn, design or document codebase by putting breadcrumbs in source code. Live updates, multi-language support and more.
https://github.com/Bogdan-Lyashenko/codecrumbs
Learn, design or document codebase by putting breadcrumbs in source code. Live updates, multi-language support and more. - Bogdan-Lyashenko/codecrumbs
- 177A functional programming library for TypeScript/JavaScript
https://github.com/marpple/FxTS
A functional programming library for TypeScript/JavaScript - marpple/FxTS
- 178🎚 HTML5 input range slider element jQuery polyfill
https://github.com/andreruffert/rangeslider.js
🎚 HTML5 input range slider element jQuery polyfill - andreruffert/rangeslider.js
- 179CSS3 backed JavaScript animation framework
https://github.com/visionmedia/move.js
CSS3 backed JavaScript animation framework. Contribute to visionmedia/move.js development by creating an account on GitHub.
- 180typeahead.js is a fast and fully-featured autocomplete library
https://github.com/twitter/typeahead.js
typeahead.js is a fast and fully-featured autocomplete library - twitter/typeahead.js
- 181React Hooks for Data Fetching
https://github.com/vercel/swr
React Hooks for Data Fetching. Contribute to vercel/swr development by creating an account on GitHub.
- 182It's a presentation framework based on the power of CSS3 transforms and transitions in modern browsers and inspired by the idea behind prezi.com.
https://github.com/impress/impress.js
It's a presentation framework based on the power of CSS3 transforms and transitions in modern browsers and inspired by the idea behind prezi.com. - impress/impress.js
- 183JSLint, The JavaScript Code Quality and Coverage Tool
https://github.com/douglascrockford/JSLint
JSLint, The JavaScript Code Quality and Coverage Tool - jslint-org/jslint
- 184Guide your users through a tour of your app
https://github.com/HubSpot/shepherd
Guide your users through a tour of your app. Contribute to shepherd-pro/shepherd development by creating an account on GitHub.
- 185blanket.js is a simple code coverage library for javascript. Designed to be easy to install and use, for both browser and nodejs.
https://github.com/alex-seville/blanket
blanket.js is a simple code coverage library for javascript. Designed to be easy to install and use, for both browser and nodejs. - alex-seville/blanket
- 186☕️ simple, flexible, fun javascript test framework for node.js & the browser
https://github.com/mochajs/mocha
☕️ simple, flexible, fun javascript test framework for node.js & the browser - mochajs/mocha
- 187Next-generation DOM manipulation
https://github.com/ractivejs/ractive
Next-generation DOM manipulation. Contribute to ractivejs/ractive development by creating an account on GitHub.
- 188kripken/sql.js
https://github.com/kripken/sql.js
Contribute to kripken/sql.js development by creating an account on GitHub.
- 189A touch slideout navigation menu for your mobile web apps.
https://github.com/mango/slideout
A touch slideout navigation menu for your mobile web apps. - Mango/slideout
- 190⏰ Day.js 2kB immutable date-time library alternative to Moment.js with the same modern API
https://github.com/iamkun/dayjs
⏰ Day.js 2kB immutable date-time library alternative to Moment.js with the same modern API - iamkun/dayjs
- 191FieldVal/fieldval-js
https://github.com/FieldVal/fieldval-js
Contribute to FieldVal/fieldval-js development by creating an account on GitHub.
- 192Lightweight and simple JS date formatting and parsing
https://github.com/taylorhakes/fecha
Lightweight and simple JS date formatting and parsing - taylorhakes/fecha
- 193A light-weight, no-dependency, vanilla JavaScript engine to drive the user's focus across the page
https://github.com/kamranahmedse/driver.js
A light-weight, no-dependency, vanilla JavaScript engine to drive the user's focus across the page - kamranahmedse/driver.js
- 194i18next: learn once - translate everywhere
https://github.com/i18next/i18next
i18next: learn once - translate everywhere. Contribute to i18next/i18next development by creating an account on GitHub.
- 195A jQuery plugin for producing big, bold & responsive headlines
https://github.com/freqDec/slabText/
A jQuery plugin for producing big, bold & responsive headlines - freqdec/slabText
- 196Buttons with built-in loading indicators.
https://github.com/hakimel/Ladda
Buttons with built-in loading indicators. Contribute to hakimel/Ladda development by creating an account on GitHub.
- 197jQuery plugin for creating interactive parallax effect
https://github.com/stephband/jparallax
jQuery plugin for creating interactive parallax effect - stephband/jparallax
- 198jQuery Hotkeys lets you watch for keyboard events anywhere in your code supporting almost any key combination.
https://github.com/jeresig/jquery.hotkeys
jQuery Hotkeys lets you watch for keyboard events anywhere in your code supporting almost any key combination. - jeresig/jquery.hotkeys
- 199Quick and easy product tours with Twitter Bootstrap Popovers
https://github.com/sorich87/bootstrap-tour
Quick and easy product tours with Twitter Bootstrap Popovers - sorich87/bootstrap-tour
- 200🤠 An opinionated AJAX client for Ruby on Rails APIs
https://github.com/victor-am/rails-ranger
🤠 An opinionated AJAX client for Ruby on Rails APIs - victor-am/rails-ranger
- 201Fraction is a rational numbers library written in JavaScript
https://github.com/infusion/Fraction.js
Fraction is a rational numbers library written in JavaScript - rawify/Fraction.js
- 202A simple micro-library for defining and dispatching keyboard shortcuts. It has no dependencies.
https://github.com/madrobby/keymaster
A simple micro-library for defining and dispatching keyboard shortcuts. It has no dependencies. - madrobby/keymaster
- 203🔮 An easy-to-use JavaScript unit testing framework.
https://github.com/jquery/qunit
🔮 An easy-to-use JavaScript unit testing framework. - qunitjs/qunit
- 204framework-agnostic styled alert system for javascript
https://github.com/hxgf/smoke.js
framework-agnostic styled alert system for javascript - jyoungblood/smoke.js
- 205a browser detector
https://github.com/ded/bowser
a browser detector. Contribute to bowser-js/bowser development by creating an account on GitHub.
- 206A Node.js CMS written in CoffeeScript, with a user friendly backend
https://github.com/nodize/nodizecms
A Node.js CMS written in CoffeeScript, with a user friendly backend - nodize/nodizecms
- 207Spectacular Test Runner for JavaScript
https://github.com/karma-runner/karma
Spectacular Test Runner for JavaScript. Contribute to karma-runner/karma development by creating an account on GitHub.
- 208Runtime type checking for JS with Hindley Milner signatures
https://github.com/xodio/hm-def
Runtime type checking for JS with Hindley Milner signatures - xodio/hm-def
- 209CasperJS is no longer actively maintained. Navigation scripting and testing utility for PhantomJS and SlimerJS
https://github.com/casperjs/casperjs
CasperJS is no longer actively maintained. Navigation scripting and testing utility for PhantomJS and SlimerJS - casperjs/casperjs
- 210High-level API for working with binary data.
https://github.com/jDataView/jBinary
High-level API for working with binary data. Contribute to jDataView/jBinary development by creating an account on GitHub.
- 211Smarter defaults for colors on the web.
https://github.com/mrmrs/colors
Smarter defaults for colors on the web. Contribute to mrmrs/colors development by creating an account on GitHub.
- 212A reactive atomic state engine for React like.
https://github.com/concentjs/concent
A reactive atomic state engine for React like. Contribute to heluxjs/helux development by creating an account on GitHub.
- 213Javascript URL mutation library
https://github.com/medialize/URI.js/
Javascript URL mutation library. Contribute to medialize/URI.js development by creating an account on GitHub.
- 214⚛️ Fast 3kB React alternative with the same modern API. Components & Virtual DOM.
https://github.com/developit/preact
⚛️ Fast 3kB React alternative with the same modern API. Components & Virtual DOM. - preactjs/preact
- 215Fast and powerful CSV (delimited text) parser that gracefully handles large files and malformed input
https://github.com/mholt/PapaParse
Fast and powerful CSV (delimited text) parser that gracefully handles large files and malformed input - mholt/PapaParse
- 216JQuery powered parallaxing
https://github.com/cameronmcefee/plax
JQuery powered parallaxing. Contribute to cameronmcefee/plax development by creating an account on GitHub.
- 217db.js is a wrapper for IndexedDB to make it easier to work against
https://github.com/aaronpowell/db.js/
db.js is a wrapper for IndexedDB to make it easier to work against - aaronpowell/db.js
- 218add some slide effects.
https://github.com/bchanx/slidr
add some slide effects. Contribute to bchanx/slidr development by creating an account on GitHub.
- 219This Is Responsive
https://github.com/bradfrost/this-is-responsive
This Is Responsive. Contribute to bradfrost/this-is-responsive development by creating an account on GitHub.
- 220Javascript library to create physics-based animations
https://github.com/michaelvillar/dynamics.js
Javascript library to create physics-based animations - michaelvillar/dynamics.js
- 221A JavaScript Framework for Building Brilliant Applications
https://github.com/lhorie/mithril.js
A JavaScript Framework for Building Brilliant Applications - MithrilJS/mithril.js
- 222Library for interactive videos in React
https://github.com/ysulyma/ractive-player
Library for interactive videos in React. Contribute to liqvidjs/liqvid development by creating an account on GitHub.
- 223🛤 Detection of elements in viewport & smooth scrolling with parallax.
https://github.com/locomotivemtl/locomotive-scroll
🛤 Detection of elements in viewport & smooth scrolling with parallax. - locomotivemtl/locomotive-scroll
- 224🔮 Proxies nodejs require in order to allow overriding dependencies during testing.
https://github.com/thlorenz/proxyquire
🔮 Proxies nodejs require in order to allow overriding dependencies during testing. - thlorenz/proxyquire
- 225Pug – robust, elegant, feature rich template engine for Node.js
https://github.com/pugjs/pug
Pug – robust, elegant, feature rich template engine for Node.js - pugjs/pug
- 226JavaScript 3D Library.
https://github.com/mrdoob/three.js
JavaScript 3D Library. Contribute to mrdoob/three.js development by creating an account on GitHub.
- 227This is the repo for Vue 2. For Vue 3, go to https://github.com/vuejs/core
https://github.com/vuejs/vue
This is the repo for Vue 2. For Vue 3, go to https://github.com/vuejs/core - vuejs/vue
- 228simplified jsdoc 3
https://github.com/sutoiku/jsdox
simplified jsdoc 3. Contribute to sutoiku/jsdox development by creating an account on GitHub.
- 229:kangaroo: - PouchDB is a pocket-sized database.
https://github.com/pouchdb/pouchdb
:kangaroo: - PouchDB is a pocket-sized database. Contribute to pouchdb/pouchdb development by creating an account on GitHub.
- 230💾 Offline storage, improved. Wraps IndexedDB, WebSQL, or localStorage using a simple but powerful API.
https://github.com/mozilla/localForage
💾 Offline storage, improved. Wraps IndexedDB, WebSQL, or localStorage using a simple but powerful API. - localForage/localForage
- 231Ace (Ajax.org Cloud9 Editor)
https://github.com/ajaxorg/ace
Ace (Ajax.org Cloud9 Editor). Contribute to ajaxorg/ace development by creating an account on GitHub.
- 232The ultimate JavaScript string library
https://github.com/panzerdp/voca
The ultimate JavaScript string library. Contribute to panzerdp/voca development by creating an account on GitHub.
- 233A web-based tool to view, edit, format, and validate JSON
https://github.com/josdejong/jsoneditor
A web-based tool to view, edit, format, and validate JSON - josdejong/jsoneditor
- 234:zap: A sliding swipe menu that works with touchSwipe library.
https://github.com/JoanClaret/slide-and-swipe-menu
:zap: A sliding swipe menu that works with touchSwipe library. - JoanClaret/slide-and-swipe-menu
- 235tap-producing test harness for node and browsers
https://github.com/substack/tape
tap-producing test harness for node and browsers. Contribute to tape-testing/tape development by creating an account on GitHub.
- 236JavaScript plugin for playing sounds and music in browsers
https://github.com/IonDen/ion.sound
JavaScript plugin for playing sounds and music in browsers - IonDen/ion.sound
- 237The fastest + concise javascript template engine for nodejs and browsers. Partials, custom delimiters and more.
https://github.com/olado/doT
The fastest + concise javascript template engine for nodejs and browsers. Partials, custom delimiters and more. - GitHub - olado/doT: The fastest + concise javascript template engine for nodejs an...
- 238A lightweight JavaScript library for number, money and currency formatting. (MOVED)
https://github.com/josscrowcroft/accounting.js
A lightweight JavaScript library for number, money and currency formatting. (MOVED) - wjcrowcroft/accounting.js
- 239JavaScript API for Chrome and Firefox
https://github.com/GoogleChrome/puppeteer
JavaScript API for Chrome and Firefox. Contribute to puppeteer/puppeteer development by creating an account on GitHub.
- 240A JQuery plugin to create AJAX based CRUD tables.
https://github.com/hikalkan/jtable
A JQuery plugin to create AJAX based CRUD tables. Contribute to volosoft/jtable development by creating an account on GitHub.
- 241Automatically add a progress bar to your site.
https://github.com/HubSpot/pace
Automatically add a progress bar to your site. Contribute to CodeByZach/pace development by creating an account on GitHub.
- 242Components for interactive scientific writing, reactive documents and explorable explanations.
https://github.com/iooxa/article
Components for interactive scientific writing, reactive documents and explorable explanations. - curvenote/article
- 243stokado can proxy objects of any storage-like, providing getter/setter syntax sugars, serialization, subscription listening, expiration setting, one-time value retrieval.
https://github.com/KID-joker/proxy-web-storage
stokado can proxy objects of any storage-like, providing getter/setter syntax sugars, serialization, subscription listening, expiration setting, one-time value retrieval. - KID-joker/stokado
- 244Easily add "zoom on hover" functionality to your site's images. Lightweight, no-dependency JavaScript.
https://github.com/imgix/drift
Easily add "zoom on hover" functionality to your site's images. Lightweight, no-dependency JavaScript. - strawdynamics/drift
- 245JavaScript package manager - using a browser-focused and RequireJS compatible repository
https://github.com/caolan/jam
JavaScript package manager - using a browser-focused and RequireJS compatible repository - caolan/jam
- 246Smooth scrolling for the web
https://github.com/cubiq/iscroll
Smooth scrolling for the web. Contribute to cubiq/iscroll development by creating an account on GitHub.
- 247Date() for humans
https://github.com/MatthewMueller/date
Date() for humans. Contribute to matthewmueller/date development by creating an account on GitHub.
- 248A reactive programming library for JavaScript
https://github.com/ReactiveX/rxjs
A reactive programming library for JavaScript. Contribute to ReactiveX/rxjs development by creating an account on GitHub.
- 249sprintf.js is a complete open source JavaScript sprintf implementation
https://github.com/alexei/sprintf.js
sprintf.js is a complete open source JavaScript sprintf implementation - alexei/sprintf.js
- 250A command line tool for translating JSON, YAML, CSV, ARB, XML (via a CLI)
https://github.com/fkirc/attranslate
A command line tool for translating JSON, YAML, CSV, ARB, XML (via a CLI) - fkirc/attranslate
- 251Lightweight URL manipulation with JavaScript
https://github.com/Mikhus/domurl
Lightweight URL manipulation with JavaScript. Contribute to Mikhus/domurl development by creating an account on GitHub.
- 252Parse, validate, manipulate, and display dates in javascript.
https://github.com/moment/moment
Parse, validate, manipulate, and display dates in javascript. - moment/moment
- 253Webplate is an awesome front-end framework that lets you stay focused on building your site or app all the while remaining really easy to use.
https://github.com/chrishumboldt/webplate
Webplate is an awesome front-end framework that lets you stay focused on building your site or app all the while remaining really easy to use. - chrishumboldt/Webplate
- 254A dependency-free JavaScript ES6 slider and carousel. It’s lightweight, flexible and fast. Designed to slide. No less, no more
https://github.com/jedrzejchalubek/glidejs
A dependency-free JavaScript ES6 slider and carousel. It’s lightweight, flexible and fast. Designed to slide. No less, no more - glidejs/glide
- 255bankfacil/vanilla-masker
https://github.com/BankFacil/vanilla-masker
Contribute to bankfacil/vanilla-masker development by creating an account on GitHub.
- 256:surfer: Asynchronous flow control with a functional taste to it
https://github.com/bevacqua/contra/
:surfer: Asynchronous flow control with a functional taste to it - bevacqua/contra
- 257DEPRECATED - A front-end template that helps you build fast, modern mobile web apps.
https://github.com/h5bp/mobile-boilerplate
DEPRECATED - A front-end template that helps you build fast, modern mobile web apps. - h5bp/mobile-boilerplate
- 258browser-side require() the node.js way
https://github.com/substack/node-browserify
browser-side require() the node.js way. Contribute to browserify/browserify development by creating an account on GitHub.
- 259A simple, lightweight JavaScript API for handling browser cookies
https://github.com/js-cookie/js-cookie
A simple, lightweight JavaScript API for handling browser cookies - js-cookie/js-cookie
- 260Pure JavaScript library for better notification messages
https://github.com/apvarun/toastify-js
Pure JavaScript library for better notification messages - apvarun/toastify-js
- 261Simple, flexible tours for your app
https://github.com/easelinc/tourist
Simple, flexible tours for your app. Contribute to easelinc/tourist development by creating an account on GitHub.
- 262A library optimized for concise and principled data graphics and layouts.
https://github.com/mozilla/metrics-graphics
A library optimized for concise and principled data graphics and layouts. - metricsgraphics/metrics-graphics
- 263String manipulation helpers for javascript
https://github.com/epeli/underscore.string
String manipulation helpers for javascript. Contribute to esamattis/underscore.string development by creating an account on GitHub.
- 264GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web
https://github.com/greensock/GreenSock-JS
GSAP (GreenSock Animation Platform), a JavaScript animation library for the modern web - greensock/GSAP
- 265Open-Source Notification Platform. Embeddable Notification Center, E-mail, Push and Slack Integrations.
https://github.com/notifirehq/notifire
Open-Source Notification Platform. Embeddable Notification Center, E-mail, Push and Slack Integrations. - novuhq/novu
- 266tsParticles - Easily create highly customizable JavaScript particles effects, confetti explosions and fireworks animations and use them as animated backgrounds for your website. Ready to use components available for React.js, Vue.js (2.x and 3.x), Angular, Svelte, jQuery, Preact, Inferno, Solid, Riot and Web Components.
https://github.com/matteobruni/tsparticles
tsParticles - Easily create highly customizable JavaScript particles effects, confetti explosions and fireworks animations and use them as animated backgrounds for your website. Ready to use compon...
- 267An async control-flow library that makes stepping through logic easy.
https://github.com/creationix/step/
An async control-flow library that makes stepping through logic easy. - creationix/step
- 268⏳ Modern JavaScript date utility library ⌛️
https://github.com/date-fns/date-fns
⏳ Modern JavaScript date utility library ⌛️. Contribute to date-fns/date-fns development by creating an account on GitHub.
- 269Multiline strings in JavaScript
https://github.com/sindresorhus/multiline
Multiline strings in JavaScript. Contribute to sindresorhus/multiline development by creating an account on GitHub.
- 270A package manager for the web
https://github.com/bower/bower
A package manager for the web. Contribute to bower/bower development by creating an account on GitHub.
- 271The world's #1 JavaScript library for rich text editing. Available for React, Vue and Angular
https://github.com/tinymce/tinymce
The world's #1 JavaScript library for rich text editing. Available for React, Vue and Angular - tinymce/tinymce
- 272Bring data to life with SVG, Canvas and HTML. :bar_chart::chart_with_upwards_trend::tada:
https://github.com/d3/d3
Bring data to life with SVG, Canvas and HTML. :bar_chart::chart_with_upwards_trend::tada: - d3/d3
- 273HashMap JavaScript class for Node.js and the browser. The keys can be anything and won't be stringified
https://github.com/flesler/hashmap
HashMap JavaScript class for Node.js and the browser. The keys can be anything and won't be stringified - flesler/hashmap
- 274Business class content management for Node.js (plugins, server cluster management, data-driven pages)
https://github.com/pencilblue/pencilblue/
Business class content management for Node.js (plugins, server cluster management, data-driven pages) - pencilblue/pencilblue
- 275ProgressJs is a JavaScript and CSS3 library which help developers to create and manage progress bar for every objects on the page.
https://github.com/usablica/progress.js
ProgressJs is a JavaScript and CSS3 library which help developers to create and manage progress bar for every objects on the page. - GitHub - usablica/progress.js: ProgressJs is a JavaScript and C...
- 276A JavaScript Quaternion library
https://github.com/infusion/Quaternion.js
A JavaScript Quaternion library. Contribute to rawify/Quaternion.js development by creating an account on GitHub.
- 277JavaScript OAuth 1.0a signature generator (RFC 5849) for node and the browser
https://github.com/bettiolo/oauth-signature-js
JavaScript OAuth 1.0a signature generator (RFC 5849) for node and the browser - bettiolo/oauth-signature-js
- 278Give your pages some headroom. Hide your header until you need it
https://github.com/WickyNilliams/headroom.js
Give your pages some headroom. Hide your header until you need it - WickyNilliams/headroom.js
- 279🚀 Strapi is the leading open-source headless CMS. It’s 100% JavaScript/TypeScript, fully customizable and developer-first.
https://github.com/strapi/strapi
🚀 Strapi is the leading open-source headless CMS. It’s 100% JavaScript/TypeScript, fully customizable and developer-first. - strapi/strapi
- 280Progressive <svg> pie, donut, bar and line charts
https://github.com/benpickles/peity
Progressive <svg> pie, donut, bar and line charts. Contribute to benpickles/peity development by creating an account on GitHub.
- 281A lightweight carousel library with fluid motion and great swipe precision.
https://github.com/davidcetinkaya/embla-carousel
A lightweight carousel library with fluid motion and great swipe precision. - davidjerleke/embla-carousel
- 282A few simple, but solid patterns for responsive HTML email templates and newsletters. Even in Outlook and Gmail.
https://github.com/TedGoas/Cerberus
A few simple, but solid patterns for responsive HTML email templates and newsletters. Even in Outlook and Gmail. - emailmonday/Cerberus
- 283HTML5 <audio> or <video> player with support for MP4, WebM, and MP3 as well as HLS, Dash, YouTube, Facebook, SoundCloud and others with a common HTML5 MediaElement API, enabling a consistent UI in all browsers.
https://github.com/johndyer/mediaelement
HTML5 <audio> or <video> player with support for MP4, WebM, and MP3 as well as HLS, Dash, YouTube, Facebook, SoundCloud and others with a common HTML5 MediaElement API, enabling a consi...
- 284An awesome, fully responsive jQuery slider plugin
https://github.com/woothemes/FlexSlider
An awesome, fully responsive jQuery slider plugin. Contribute to woocommerce/FlexSlider development by creating an account on GitHub.
- 285Raptor, an HTML5 WYSIWYG content editor!
https://github.com/PANmedia/raptor-editor
Raptor, an HTML5 WYSIWYG content editor! Contribute to PANmedia/raptor-editor development by creating an account on GitHub.
- 286Lightweight MVC library for building JavaScript applications
https://github.com/spine/spine
Lightweight MVC library for building JavaScript applications - spine/spine
- 287A blazing fast js bundler/loader with a comprehensive API :fire:
https://github.com/fuse-box/fuse-box
A blazing fast js bundler/loader with a comprehensive API :fire: - fuse-box/fuse-box
- 288Official distribution releases of CKEditor 4.
https://github.com/ckeditor/ckeditor-releases
Official distribution releases of CKEditor 4. Contribute to ckeditor/ckeditor4-releases development by creating an account on GitHub.
- 289Take a swig of the best template engine for JavaScript.
https://github.com/paularmstrong/swig
Take a swig of the best template engine for JavaScript. - paularmstrong/swig
- 290easier than regex string matching patterns for urls and other strings. turn strings into data or data into strings.
https://github.com/snd/url-pattern
easier than regex string matching patterns for urls and other strings. turn strings into data or data into strings. - snd/url-pattern
- 291Luckysheet is an online spreadsheet like excel that is powerful, simple to configure, and completely open source.
https://github.com/mengshukeji/Luckysheet
Luckysheet is an online spreadsheet like excel that is powerful, simple to configure, and completely open source. - dream-num/Luckysheet
- 292JavaScript documentation generator for node using markdown and jsdoc
https://github.com/tj/dox
JavaScript documentation generator for node using markdown and jsdoc - tj/dox
- 293A complete, fully tested and documented data structure library written in pure JavaScript.
https://github.com/mauriciosantos/Buckets-JS
A complete, fully tested and documented data structure library written in pure JavaScript. - mauriciosantos/Buckets-JS
- 294Give your JavaScript the ability to speak many languages.
https://github.com/airbnb/polyglot.js
Give your JavaScript the ability to speak many languages. - airbnb/polyglot.js
- 295:leaves: Touch, responsive, flickable carousels
https://github.com/metafizzy/flickity
:leaves: Touch, responsive, flickable carousels. Contribute to metafizzy/flickity development by creating an account on GitHub.
- 296JavaScript image gallery for mobile and desktop, modular, framework independent
https://github.com/dimsemenov/PhotoSwipe
JavaScript image gallery for mobile and desktop, modular, framework independent - dimsemenov/PhotoSwipe
- 297🦎 Move an async function into its own thread.
https://github.com/developit/greenlet
🦎 Move an async function into its own thread. Contribute to developit/greenlet development by creating an account on GitHub.
- 298Delightful JavaScript Testing.
https://github.com/facebook/jest
Delightful JavaScript Testing. Contribute to jestjs/jest development by creating an account on GitHub.
- 299A neural network library built in JavaScript
https://github.com/stevenmiller888/mind
A neural network library built in JavaScript. Contribute to stevenmiller888/mind development by creating an account on GitHub.
- 300A caffeine driven, simplistic approach to benchmarking.
https://github.com/logicalparadox/matcha
A caffeine driven, simplistic approach to benchmarking. - logicalparadox/matcha
- 301:guide_dog: Powerful lowcode|vue form editor,generator,designer,builder library. It provides an easy way to create custom forms. The project is extensible, easy to use and configure, and provides many commonly used form components and functions(vue可视化低代码表单设计器、表单编辑器、element-plus vant表单设计)
https://github.com/Liberty-liu/Everright-formEditor
:guide_dog: Powerful lowcode|vue form editor,generator,designer,builder library. It provides an easy way to create custom forms. The project is extensible, easy to use and configure, and provides m...
- 302Resize image in browser with high quality and high speed
https://github.com/nodeca/pica
Resize image in browser with high quality and high speed - nodeca/pica
- 303(Public Release Summer 2024) Personal Marketing Platform. A powerful platform for your online identity.
https://github.com/fiction-com/factor
(Public Release Summer 2024) Personal Marketing Platform. A powerful platform for your online identity. - fictionco/fiction
- 304Dynamic Neural Networks Architect
https://github.com/dn2a/dn2a-javascript
Dynamic Neural Networks Architect. Contribute to antoniodeluca/dn2a development by creating an account on GitHub.
- 305A datepicker for twitter bootstrap (@twbs)
https://github.com/eternicode/bootstrap-datepicker
A datepicker for twitter bootstrap (@twbs). Contribute to uxsolutions/bootstrap-datepicker development by creating an account on GitHub.
- 306Easy Parallax Effect for React & JavaScript
https://github.com/geosigno/simpleParallax
Easy Parallax Effect for React & JavaScript. Contribute to geosigno/simpleParallax.js development by creating an account on GitHub.
- 307String validation
https://github.com/chriso/validator.js
String validation. Contribute to validatorjs/validator.js development by creating an account on GitHub.
- 308A refreshing JavaScript Datepicker — lightweight, no dependencies, modular CSS
https://github.com/dbushell/Pikaday
A refreshing JavaScript Datepicker — lightweight, no dependencies, modular CSS - Pikaday/Pikaday
- 309Brand new static package manager.
https://github.com/spmjs/spm
Brand new static package manager. Contribute to spmjs/spm development by creating an account on GitHub.
- 310The library which provides useful monads, interfaces, and lazy iterators.
https://github.com/JSMonk/sweet-monads
The library which provides useful monads, interfaces, and lazy iterators. - JSMonk/sweet-monads
- 311Deliver web apps with confidence 🚀
https://github.com/angular/angular
Deliver web apps with confidence 🚀. Contribute to angular/angular development by creating an account on GitHub.
- 312money.js is a tiny (1kb) javascript currency conversion library, for web & nodeJS. (MOVED)
https://github.com/josscrowcroft/money.js
money.js is a tiny (1kb) javascript currency conversion library, for web & nodeJS. (MOVED) - wjcrowcroft/money.js
- 313Cross domain local storage, with permissions
https://github.com/zendesk/cross-storage
Cross domain local storage, with permissions. Contribute to zendesk/cross-storage development by creating an account on GitHub.
- 314Complex.js is a com numbers library written in JavaScript
https://github.com/infusion/Complex.js
Complex.js is a com numbers library written in JavaScript - infusion/Complex.js
- 315A script and resource loader for caching & loading files with localStorage
https://github.com/addyosmani/basket.js
A script and resource loader for caching & loading files with localStorage - addyosmani/basket.js
- 316Emulate touch input on your desktop
https://github.com/hammerjs/touchemulator
Emulate touch input on your desktop. Contribute to hammerjs/touchemulator development by creating an account on GitHub.
- 317Material Progress —Google Material Design Progress linear bar. By using CSS3 and vanilla JavaScript.
https://github.com/lightningtgc/MProgress.js
Material Progress —Google Material Design Progress linear bar. By using CSS3 and vanilla JavaScript. - GitHub - lightningtgc/MProgress.js: Material Progress —Google Material Design Progress linear...
- 318🔥 Static site generator for Deno 🦕
https://github.com/lumeland/lume
🔥 Static site generator for Deno 🦕. Contribute to lumeland/lume development by creating an account on GitHub.
- 319The best javascript plugin for app look-alike on- and off-canvas menus with sliding submenus for your website and webapp.
https://github.com/FrDH/jQuery.mmenu
The best javascript plugin for app look-alike on- and off-canvas menus with sliding submenus for your website and webapp. - FrDH/mmenu-js
- 320A Node.js tool to automate end-to-end web testing.
https://github.com/DevExpress/testcafe
A Node.js tool to automate end-to-end web testing. - DevExpress/testcafe
- 321🙋♀️ 3kb library for tiny web apps
https://github.com/aidenybai/lucia
🙋♀️ 3kb library for tiny web apps. Contribute to aidenybai/lucia development by creating an account on GitHub.
- 322A jQuery plugin for easy consumption of RESTful APIs
https://github.com/jpillora/jquery.rest
A jQuery plugin for easy consumption of RESTful APIs - jpillora/jquery.rest
- 323Detect copy-pasted and structurally similar code
https://github.com/danielstjules/jsinspect
Detect copy-pasted and structurally similar code. Contribute to danielstjules/jsinspect development by creating an account on GitHub.
- 324Full-stack CRUD, simplified, with SSOT TypeScript entities
https://github.com/remult/remult
Full-stack CRUD, simplified, with SSOT TypeScript entities - remult/remult
- 325You're looking for https://github.com/rpominov/kefir
https://github.com/pozadi/kefir
You're looking for https://github.com/rpominov/kefir - pozadi/kefir
- 326A framework for building native applications using React
https://github.com/facebook/react-native
A framework for building native applications using React - facebook/react-native
- 327Test runner based on Tape and Browserify
https://github.com/azer/prova
Test runner based on Tape and Browserify. Contribute to azer/prova development by creating an account on GitHub.
- 328Super minimal MVC library
https://github.com/techlayer/espresso.js
Super minimal MVC library. Contribute to akrymski/espresso.js development by creating an account on GitHub.
- 329Smoothly transitions numbers with ease. #hubspot-open-source
https://github.com/HubSpot/odometer
Smoothly transitions numbers with ease. #hubspot-open-source - HubSpot/odometer
- 330Lightweight JavaScript module system.
https://github.com/lrsjng/modulejs
Lightweight JavaScript module system. Contribute to lrsjng/modulejs development by creating an account on GitHub.
- 331Super fast spec-compliant URL state machine for Node.js
https://github.com/anonrig/url-js
Super fast spec-compliant URL state machine for Node.js - anonrig/url-js
- 332JS / CSS / files loader + key/value storage
https://github.com/nodeca/bag.js
JS / CSS / files loader + key/value storage. Contribute to nodeca/bag.js development by creating an account on GitHub.
- 333JavaScript port of Vim
https://github.com/coolwanglu/vim.js
JavaScript port of Vim. Contribute to coolwanglu/vim.js development by creating an account on GitHub.
- 334:dango: An interactive and responsive charting library based on G2.
https://github.com/antvis/G2Plot
:dango: An interactive and responsive charting library based on G2. - antvis/G2Plot
- 335A JavaScript library to work with polynomials
https://github.com/infusion/Polynomial.js
A JavaScript library to work with polynomials. Contribute to infusion/Polynomial.js development by creating an account on GitHub.
- 336A Javascript library for working with native objects.
https://github.com/andrewplummer/Sugar
A Javascript library for working with native objects. - andrewplummer/Sugar
- 337The mobile-friendly, responsive, and lightweight jQuery date & time input picker.
https://github.com/amsul/pickadate.js
The mobile-friendly, responsive, and lightweight jQuery date & time input picker. - amsul/pickadate.js
- 338Ultra-high performance reactive programming
https://github.com/cujojs/most
Ultra-high performance reactive programming. Contribute to cujojs/most development by creating an account on GitHub.
- 339The web-based visual programming editor.
https://github.com/google/blockly
The web-based visual programming editor. Contribute to google/blockly development by creating an account on GitHub.
- 340A collection of loading indicators animated with CSS
https://github.com/tobiasahlin/SpinKit
A collection of loading indicators animated with CSS - tobiasahlin/SpinKit
- 341UNMAINTAINED Open source JavaScript renderer for Kartograph SVG maps
https://github.com/kartograph/kartograph.js
UNMAINTAINED Open source JavaScript renderer for Kartograph SVG maps - kartograph/kartograph.js
- 342Create beautiful CSS3 powered animations in no time.
https://github.com/tictail/bounce.js
Create beautiful CSS3 powered animations in no time. - tictail/bounce.js
- 3431kB-ish JavaScript framework for building hypertext applications
https://github.com/hyperapp/hyperapp
1kB-ish JavaScript framework for building hypertext applications - jorgebucaran/hyperapp
- 344Simple wrapper for cross-browser usage of the JavaScript Fullscreen API
https://github.com/sindresorhus/screenfull.js
Simple wrapper for cross-browser usage of the JavaScript Fullscreen API - sindresorhus/screenfull
- 345JavaScript toolkit for creating interactive real-time graphs
https://github.com/shutterstock/rickshaw
JavaScript toolkit for creating interactive real-time graphs - shutterstock/rickshaw
- 346Plain functions for a more functional Deku approach to creating stateless React components, with functional goodies such as compose, memoize, etc... for free.
https://github.com/Wildhoney/Keo
Plain functions for a more functional Deku approach to creating stateless React components, with functional goodies such as compose, memoize, etc... for free. - Wildhoney/Keo
- 347The smallest, most flexible online video player - Wowza Flowplayer
https://flowplayer.com/
Customisable, fast and powerful video player & platform. With 36 different player plugins and complete with mobile SDKs. Simple to deploy. Trusted by thousands.
- 348A next-generation code testing stack for JavaScript.
https://github.com/theintern/intern
A next-generation code testing stack for JavaScript. - theintern/intern
- 349Next-generation ES module bundler
https://github.com/rollup/rollup
Next-generation ES module bundler. Contribute to rollup/rollup development by creating an account on GitHub.
- 350Simple and elegant component-based UI library
https://github.com/riot/riot
Simple and elegant component-based UI library. Contribute to riot/riot development by creating an account on GitHub.
- 351Open source rich text editor based on HTML5 and the progressive-enhancement approach. Uses a sophisticated security concept and aims to generate fully valid HTML5 markup by preventing unmaintainable tag soups and inline styles.
https://github.com/xing/wysihtml5
Open source rich text editor based on HTML5 and the progressive-enhancement approach. Uses a sophisticated security concept and aims to generate fully valid HTML5 markup by preventing unmaintainabl...
- 352jStorage is a simple key/value database to store data on browser side
https://github.com/andris9/jStorage
jStorage is a simple key/value database to store data on browser side - andris9/jStorage
- 353:bird: Bluebird is a full featured promise library with unmatched performance.
https://github.com/petkaantonov/bluebird/
:bird: :zap: Bluebird is a full featured promise library with unmatched performance. - GitHub - petkaantonov/bluebird: :bird: Bluebird is a full featured promise library with unmatched performance.
- 354Async utilities for node and the browser
https://github.com/caolan/async
Async utilities for node and the browser. Contribute to caolan/async development by creating an account on GitHub.
- 355Extract prominent colors from an image. JS port of Android's Palette.
https://github.com/jariz/vibrant.js/
Extract prominent colors from an image. JS port of Android's Palette. - jariz/vibrant.js
- 356Like Underscore, but lazier
https://github.com/dtao/lazy.js
Like Underscore, but lazier. Contribute to dtao/lazy.js development by creating an account on GitHub.
- 357:orange_book: simple approach for javascript localization
https://github.com/ttag-org/ttag
:orange_book: simple approach for javascript localization - ttag-org/ttag
- 358Make your text sizing responsive!
https://github.com/ghepting/jquery-responsive-text
Make your text sizing responsive! Contribute to ghepting/jquery-responsive-text development by creating an account on GitHub.
- 359JavaScript library for one-directional scrolling with item based navigation support.
https://github.com/darsain/sly
JavaScript library for one-directional scrolling with item based navigation support. - darsain/sly
- 360The responsive CSS animation framework for creating unique sliders, presentations, banners, and other step-based applications.
https://github.com/IanLunn/Sequence
The responsive CSS animation framework for creating unique sliders, presentations, banners, and other step-based applications. - IanLunn/Sequence
- 361Client-side JavaScript PDF generation for everyone.
https://github.com/MrRio/jsPDF
Client-side JavaScript PDF generation for everyone. - parallax/jsPDF
- 362A declarative, HTML-based language that makes building web apps fun
https://github.com/marko-js/marko
A declarative, HTML-based language that makes building web apps fun - marko-js/marko
- 363JavaScript syntax highlighter with language auto-detection and zero dependencies.
https://github.com/isagalaev/highlight.js
JavaScript syntax highlighter with language auto-detection and zero dependencies. - highlightjs/highlight.js
- 364The lightweight library for manipulating and animating SVG
https://github.com/wout/svg.js
The lightweight library for manipulating and animating SVG - svgdotjs/svg.js
- 365Secure XSS Filters.
https://github.com/yahoo/xss-filters
Secure XSS Filters. Contribute to YahooArchive/xss-filters development by creating an account on GitHub.
- 366Yet another JS code coverage tool that computes statement, line, function and branch coverage with module loader hooks to transparently add coverage when running tests. Supports all JS coverage use cases including unit tests, server side functional tests and browser tests. Built for scale.
https://github.com/gotwarlost/istanbul
Yet another JS code coverage tool that computes statement, line, function and branch coverage with module loader hooks to transparently add coverage when running tests. Supports all JS coverage use...
- 367Meteor, the JavaScript App Platform
https://github.com/meteor/meteor
Meteor, the JavaScript App Platform. Contribute to meteor/meteor development by creating an account on GitHub.
- 368Hatch.js - not officially supported
https://github.com/inventures/hatchjs
Hatch.js - not officially supported. Contribute to inventures/hatchjs development by creating an account on GitHub.
- 369Mailchimp Open Commerce is an API-first, headless commerce platform built using Node.js, React, GraphQL. Deployed via Docker and Kubernetes.
https://github.com/reactioncommerce/reaction
Mailchimp Open Commerce is an API-first, headless commerce platform built using Node.js, React, GraphQL. Deployed via Docker and Kubernetes. - GitHub - reactioncommerce/reaction: Mailchimp Open Co...
- 370JavaScript's utility _ belt
https://github.com/jashkenas/underscore
JavaScript's utility _ belt. Contribute to jashkenas/underscore development by creating an account on GitHub.
- 371A modern, simple and elegant WYSIWYG rich text editor.
https://github.com/raphaelcruzeiro/jquery-notebook
A modern, simple and elegant WYSIWYG rich text editor. - raphaelcruzeiro/jquery-notebook
- 372eXtensible Template Engine lib for node and the browser
https://github.com/xtemplate/xtemplate
eXtensible Template Engine lib for node and the browser - xtemplate/xtemplate
- 373A React framework for building text editors.
https://github.com/facebook/draft-js
A React framework for building text editors. Contribute to facebookarchive/draft-js development by creating an account on GitHub.
- 374vis.js
https://github.com/visjs
Dynamic, browser based visualization libraries. vis.js has 16 repositories available. Follow their code on GitHub.
- 375A group of neural-network libraries for functional and mainstream languages
https://github.com/mrdimosthenis/Synapses
A group of neural-network libraries for functional and mainstream languages - mrdimosthenis/Synapses
- 376A benchmarking library. As used on jsPerf.com.
https://github.com/bestiejs/benchmark.js
A benchmarking library. As used on jsPerf.com. Contribute to bestiejs/benchmark.js development by creating an account on GitHub.
- 377A rich text editor for everyday writing
https://github.com/basecamp/trix
A rich text editor for everyday writing. Contribute to basecamp/trix development by creating an account on GitHub.
- 378A collection of loading spinners animated with CSS
https://github.com/lukehaas/css-loaders
A collection of loading spinners animated with CSS - lukehaas/css-loaders
- 379Responsive and slick progress bars
https://github.com/kimmobrunfeldt/progressbar.js
Responsive and slick progress bars . Contribute to kimmobrunfeldt/progressbar.js development by creating an account on GitHub.
- 380:snowboarder: A responsive slider jQuery plugin with CSS animations
https://github.com/JoanClaret/jcSlider
:snowboarder: A responsive slider jQuery plugin with CSS animations - JoanClaret/jcSlider
- 381A framework to make it easy for developers to add product tours to their pages.
https://github.com/linkedin/hopscotch
A framework to make it easy for developers to add product tours to their pages. - LinkedInAttic/hopscotch
- 382Hooks for fetching, caching and updating asynchronous data in Vue
https://github.com/DamianOsipiuk/vue-query
Hooks for fetching, caching and updating asynchronous data in Vue - DamianOsipiuk/vue-query
- 383A compiler for the Mustache templating language
https://github.com/twitter/hogan.js
A compiler for the Mustache templating language. Contribute to twitter/hogan.js development by creating an account on GitHub.
- 384Clooney is an actor library for the web. Use workers without thinking about workers.
https://github.com/GoogleChromeLabs/clooney
Clooney is an actor library for the web. Use workers without thinking about workers. - GoogleChromeLabs/clooney
- 385🐐 Simple and complete React DOM testing utilities that encourage good testing practices.
https://github.com/kentcdodds/react-testing-library
🐐 Simple and complete React DOM testing utilities that encourage good testing practices. - testing-library/react-testing-library
- 386Testing Frameworks for Javascript | Write, Run, Debug | Cypress
https://www.cypress.io/
Simplify front-end testing with Cypress' open-source app. Explore our versatile testing frameworks for browser-based applications and components.
- 387High-Performance JavaScript Charts | WebGL JS Charts Library
https://www.arction.com/lightningchart-js/.
Fastest High-Performing JavaScript charts on the market. WebGL JS Charts Library with outstanding performance.
- 388Elegant, responsive, flexible and lightweight notification plugin with no dependencies.
https://github.com/dolce/iziToast
Elegant, responsive, flexible and lightweight notification plugin with no dependencies. - marcelodolza/iziToast
- 389jQuery Validation Plugin library sources
https://github.com/jzaefferer/jquery-validation
jQuery Validation Plugin library sources. Contribute to jquery-validation/jquery-validation development by creating an account on GitHub.
- 390🍿 A cross-browser library of CSS animations. As easy to use as an easy thing.
https://github.com/daneden/animate.css
🍿 A cross-browser library of CSS animations. As easy to use as an easy thing. - animate-css/animate.css
- 391A graph node engine and editor written in Javascript similar to PD or UDK Blueprints, comes with its own editor in HTML5 Canvas2D. The engine can run client side or server side using Node. It allows to export graphs as JSONs to be included in applications independently.
https://github.com/jagenjo/litegraph.js
A graph node engine and editor written in Javascript similar to PD or UDK Blueprints, comes with its own editor in HTML5 Canvas2D. The engine can run client side or server side using Node. It allow...
- 392Data Apps for Production | Plotly
https://plotly.com/
Discover data applications for production with Plotly Dash. Put data and AI into action with scalable, interactive data apps for your organization.
- 393Super simple WYSIWYG editor
https://github.com/summernote/summernote
Super simple WYSIWYG editor. Contribute to summernote/summernote development by creating an account on GitHub.
- 394Display the countdown on top of the Google Maps
https://github.com/dawidjaniga/map-countdown
Display the countdown on top of the Google Maps. Contribute to dawidjaniga/map-countdown development by creating an account on GitHub.
- 395Cross-browser storage for all use cases, used across the web.
https://github.com/marcuswestin/store.js
Cross-browser storage for all use cases, used across the web. - marcuswestin/store.js
- 396HTML as Template Language
https://github.com/Guseyn/EHTML
HTML as Template Language. Contribute to Guseyn/EHTML development by creating an account on GitHub.
- 397Lightweight, user-friendly onboarding tour library
https://github.com/usablica/intro.js
Lightweight, user-friendly onboarding tour library - usablica/intro.js
- 398jQuery plugin, calculates the font-size and word-spacing needed to match a line of text to a specific width.
https://github.com/zachleat/BigText
jQuery plugin, calculates the font-size and word-spacing needed to match a line of text to a specific width. - zachleat/BigText
- 399An open-source JavaScript library for world-class 3D globes and maps :earth_americas:
https://github.com/AnalyticalGraphicsInc/cesium
An open-source JavaScript library for world-class 3D globes and maps :earth_americas: - CesiumGS/cesium
- 400Modern Fetch API wrapper for simplicity.
https://github.com/WebsiteBeaver/far-fetch
Modern Fetch API wrapper for simplicity. Contribute to D-Marc1/far-fetch development by creating an account on GitHub.
- 401ESDoc - Good Documentation for JavaScript
https://github.com/esdoc/esdoc
ESDoc - Good Documentation for JavaScript. Contribute to esdoc/esdoc development by creating an account on GitHub.
- 402:fishing_pole_and_fish: A library that allows you to access the text selected by the user
https://github.com/EvandroLG/selecting
:fishing_pole_and_fish: A library that allows you to access the text selected by the user - EvandroLG/selecting
- 403How to add multi-threading in JS
https://www.loginradius.com/blog/async/adding-multi-threading-to-javascript-using-web-workers/
Learn how web workers help with the success of the web app and get started by creating a simple web worker for JavaScript.
- 404A full-featured, open-source content management framework built with Node.js that empowers organizations by combining in-context editing and headless architecture in a full-stack JS environment.
https://github.com/punkave/apostrophe
A full-featured, open-source content management framework built with Node.js that empowers organizations by combining in-context editing and headless architecture in a full-stack JS environment. - ...
- 405enjoy live editing (+markdown)
https://github.com/sofish/pen
enjoy live editing (+markdown). Contribute to sofish/pen development by creating an account on GitHub.
- 406Relocate resource intensive third-party scripts off of the main thread and into a web worker. 🎉
https://github.com/BuilderIO/partytown
Relocate resource intensive third-party scripts off of the main thread and into a web worker. 🎉 - BuilderIO/partytown
- 407A JavaScript polyfill for the HTML5 placeholder attribute
https://github.com/jamesallardice/Placeholders.js
A JavaScript polyfill for the HTML5 placeholder attribute - jamesallardice/Placeholders.js
- 408Makes typing in input fields fun with CSS3 effects
https://github.com/yairEO/fancyInput
Makes typing in input fields fun with CSS3 effects - yairEO/fancyInput
- 409React
https://reactjs.org/
React is the library for web and native user interfaces. Build user interfaces out of individual pieces called components written in JavaScript. React is designed to let you seamlessly combine components written by independent people, teams, and organizations.
- 410npm run-scripts boilerplate
https://gist.github.com/addyosmani/9f10c555e32a8d06ddb0
npm run-scripts boilerplate. GitHub Gist: instantly share code, notes, and snippets.
- 411A javascript library for formatting and manipulating numbers.
https://github.com/adamwdraper/Numeral-js
A javascript library for formatting and manipulating numbers. - adamwdraper/Numeral-js
- 412Home - Slide SJS
http://www.slidesjs.com
// Best IT Solution provider // Extension Development Get In Touch Learn More // Best IT Solution provider // Plugins Development Get In Touch Learn More //about us Slide SJS is a trendy IT production studio. Call Contact Centre +13605796589 if you, or someone close to you. Celestine Spencer The Company The Team Mission and […]
- 413⌨ Awesome handling of keyboard events
https://github.com/keithamus/jwerty
⌨ Awesome handling of keyboard events. Contribute to keithamus/jwerty development by creating an account on GitHub.
- 414A keyboard input capturing utility in which any key can be a modifier key.
https://github.com/dmauro/Keypress
A keyboard input capturing utility in which any key can be a modifier key. - dmauro/Keypress
- 415One property, two values, endless possiblities
https://github.com/LeaVerou/animatable
One property, two values, endless possiblities. Contribute to LeaVerou/animatable development by creating an account on GitHub.
- 416OpenAPI linting, diffing and testing. Optic helps prevent breaking changes, publish accurate documentation and improve the design of your APIs.
https://github.com/opticdev/optic
OpenAPI linting, diffing and testing. Optic helps prevent breaking changes, publish accurate documentation and improve the design of your APIs. - opticdev/optic
- 417Transform ML models into a native code (Java, C, Python, Go, JavaScript, Visual Basic, C#, R, PowerShell, PHP, Dart, Haskell, Ruby, F#, Rust) with zero dependencies
https://github.com/BayesWitnesses/m2cgen
Transform ML models into a native code (Java, C, Python, Go, JavaScript, Visual Basic, C#, R, PowerShell, PHP, Dart, Haskell, Ruby, F#, Rust) with zero dependencies - BayesWitnesses/m2cgen
- 418🧵 Make web workers & worker threads as simple as a function call.
https://github.com/andywer/threads.js
🧵 Make web workers & worker threads as simple as a function call. - andywer/threads.js
- 419🤖 Powerful asynchronous state management, server-state utilities and data fetching for the web. TS/JS, React Query, Solid Query, Svelte Query and Vue Query.
https://github.com/tannerlinsley/react-query
🤖 Powerful asynchronous state management, server-state utilities and data fetching for the web. TS/JS, React Query, Solid Query, Svelte Query and Vue Query. - TanStack/query
- 420A jQuery tooltip plugin
https://github.com/iamceege/tooltipster
A jQuery tooltip plugin. Contribute to calebjacob/tooltipster development by creating an account on GitHub.
- 421Javascript Canvas Library, SVG-to-Canvas (& canvas-to-SVG) Parser
https://github.com/kangax/fabric.js
Javascript Canvas Library, SVG-to-Canvas (& canvas-to-SVG) Parser - fabricjs/fabric.js
- 422jQuery Vector Map Library
https://github.com/manifestinteractive/jqvmap
jQuery Vector Map Library. Contribute to 10bestdesign/jqvmap development by creating an account on GitHub.
- 423A friendly reusable charts DSL for D3
https://github.com/heavysixer/d4
A friendly reusable charts DSL for D3. Contribute to heavysixer/d4 development by creating an account on GitHub.
- 424A beautiful way to read documentation
https://github.com/beautiful-docs/beautiful-docs
A beautiful way to read documentation. Contribute to beautiful-docs/beautiful-docs development by creating an account on GitHub.
- 425:clock8: The original jQuery plugin that makes it easy to support automatically updating fuzzy timestamps (e.g. "4 minutes ago").
https://github.com/rmm5t/jquery-timeago
:clock8: The original jQuery plugin that makes it easy to support automatically updating fuzzy timestamps (e.g. "4 minutes ago"). - rmm5t/jquery-timeago
- 426Knockout makes it easier to create rich, responsive UIs with JavaScript
https://github.com/knockout/knockout
Knockout makes it easier to create rich, responsive UIs with JavaScript - knockout/knockout
- 427Tiny millisecond conversion utility
https://github.com/rauchg/ms.js
Tiny millisecond conversion utility. Contribute to vercel/ms development by creating an account on GitHub.
- 428Simple, scalable state management.
https://github.com/mobxjs/mobx
Simple, scalable state management. Contribute to mobxjs/mobx development by creating an account on GitHub.
- 429A spinning activity indicator
https://github.com/fgnass/spin.js
A spinning activity indicator. Contribute to fgnass/spin.js development by creating an account on GitHub.
- 430ECMAScript 6: Feature Overview & Comparison
https://github.com/rse/es6-features
ECMAScript 6: Feature Overview & Comparison. Contribute to rse/es6-features development by creating an account on GitHub.
- 431Plupload is JavaScript API for building file uploaders. It supports multiple file selection, file filtering, chunked upload, client side image downsizing and when necessary can fallback to alternative runtimes, like Flash and Silverlight.
https://github.com/moxiecode/plupload
Plupload is JavaScript API for building file uploaders. It supports multiple file selection, file filtering, chunked upload, client side image downsizing and when necessary can fallback to alternat...
- 432Text Neon Golden effect jQuery plug-in
https://github.com/chuckyglitch/novacancy.js
Text Neon Golden effect jQuery plug-in. Contribute to chuckyglitch/novacancy.js development by creating an account on GitHub.
- 433The Simplest Way to shuffle through images in a Creative Way
https://github.com/peachananr/shuffle-images
The Simplest Way to shuffle through images in a Creative Way - peachananr/shuffle-images
- 434Prettier embeds for your YouTubes - with nice options like high-res preview images, advanced customization of embed options, and optional FitVids support.
https://github.com/mike-zarandona/prettyembed.js
Prettier embeds for your YouTubes - with nice options like high-res preview images, advanced customization of embed options, and optional FitVids support. - mike-zarandona/PrettyEmbed.js
- 435Animated typing in ~400 bytes 🐡 of JavaScript
https://github.com/camwiegert/typical
Animated typing in ~400 bytes 🐡 of JavaScript. Contribute to camwiegert/typical development by creating an account on GitHub.
- 436Rule YouTube, Soundcloud and Vimeo player with one API
https://github.com/Acconut/polyplayer
Rule YouTube, Soundcloud and Vimeo player with one API - Acconut/polyplayer
- 437Immutable persistent data collections for Javascript which increase efficiency and simplicity.
https://github.com/facebook/immutable-js
Immutable persistent data collections for Javascript which increase efficiency and simplicity. - immutable-js/immutable-js
- 438Dropzone is an easy to use drag'n'drop library. It supports image previews and shows nice progress bars.
https://github.com/enyo/dropzone
Dropzone is an easy to use drag'n'drop library. It supports image previews and shows nice progress bars. - dropzone/dropzone
- 439JavaScript emoji picker. Any app, any framework.
https://github.com/joeattardi/emoji-button
JavaScript emoji picker. Any app, any framework. Contribute to joeattardi/picmo development by creating an account on GitHub.
- 440🦩 Joi like validations for TypeScript
https://github.com/neuledge/funval
🦩 Joi like validations for TypeScript. Contribute to neuledge/computed-types development by creating an account on GitHub.
Related Articlesto learn about angular.
- 1JavaScript Basics
- 2Top 10 JavaScript Interview Questions for Beginners
- 3Understanding JavaScript Closures
- 4Mastering JavaScript Promises for Asynchronous Programming
- 5React vs Vue: Which JavaScript Framework Should You Choose?
- 6Top 5 JavaScript Libraries Every Developer Should Know
- 7Building a To-Do List App with Vanilla JavaScript
- 8How to Integrate APIs in JavaScript
- 9The Evolution of JavaScript
- 10JavaScript Debugging Techniques Every Developer Should Know
FAQ'sto learn more about Angular JS.
mail [email protected] to add more queries here 🔍.
- 1
does javascript use semicolons
- 2
are javascript objects hash tables
- 3
are javascript objects ordered
- 4
does javascript have a future
- 5
is javascript compiled or interpreted
- 6
will javascript ever be multithreaded
- 7
is javascript case sensitive
- 8
what is () = in javascript
- 9
who made javascript
- 10
which javascript framework is most popular
- 11
are javascript maps ordered
- 12
why was javascript used
- 13
are javascript and python similar
- 14
when javascript was created
- 15
are javascript and java the same
- 16
why javascript is single threaded
- 17
which javascript library is best
- 18
how javascript event loop works
- 19
what would javascript do
- 20
is javascript single threaded
- 21
do javascript functions need semicolons
- 22
how javascript works pdf
- 23
where javascript array
- 24
are javascript and typescript the same
- 25
what javascript statement in place of
- 26
do javascript postback
- 27
where javascript is used in html
- 28
when javascript loop
- 29
who maintains javascript
- 30
how javascript code is executed
- 31
will javascript replace python
- 32
how to do javascript in html
- 33
how javascript engine works
- 34
should javascript be in head or body
- 35
should javascript files be capitalized
- 36
who is the father of javascript
- 37
is javascript easy to learn
- 38
should javascript be enabled
- 39
is javascript hard to learn
- 40
what javascript developer do
- 41
who uses javascript
- 42
are javascript strings immutable
- 43
why javascript is bad
- 44
how would you initialize the variables in javascript
- 45
do while javascript example
- 46
which javascript version should i use
- 47
why javascript is not working in html
- 48
who created javascript programming language
- 49
how did javascript get its name
- 50
how javascript promises work
- 51
when javascript onload= event is detected
- 52
which javascript framework is easy to learn
- 53
can javascript be used for backend
- 54
is javascript old
- 55
who is the developer of javascript
- 56
where javascript is written
- 57
is javascript the same as java
- 58
are javascript arrays mutable
- 59
what javascript does
- 60
how long would it take to learn javascript
- 61
why javascript is used
- 62
is javascript free
- 63
what javascript do
- 64
should javascript syntax
- 65
why javascript is so popular
- 66
what javascript looks like
- 67
where javascript came from
- 68
do javascript classes need constructors
- 69
which javascript code can be written in
- 70
will javascript be replaced by ai
- 71
does safari have javascript
- 72
does javascript have tuples
- 73
what javascript can be used for
- 74
does chrome have javascript
- 75
will javascript get types
- 76
can javascript read a text file
- 77
does javascript have enums
- 78
will javascript replace vba
- 79
what javascript framework should i use
- 80
do javascript applescript
- 81
why javascript is hated
- 82
will javascript ever be replaced
- 83
will javascript become typescript
- 84
does javascript pass objects by reference
- 85
will javascript die
- 86
what javascript can do
- 87
does javascript have interfaces
- 88
when javascript invented
- 89
does leetcode have javascript
- 90
how to check javascript ad blockers
- 91
why javascript is better than python
- 92
are javascript arrays dynamic
- 93
is javascript still used
- 94
should javascript functions be capitalized
- 95
where javascript function
- 96
can javascript be used for ai
- 97
where javascript runs
- 98
do javascript objects maintain order
- 99
what javascript is used for
- 100
how to have javascript enabled
- 101
can javascript be compiled
- 102
when was javascript released
- 103
which javascript object works with the dates
- 104
how javascript works behind the scenes
- 105
why javascript is the best programming language
- 106
will javascript become obsolete
- 107
where javascript in html
- 108
can javascript write to a file
- 109
will javascript be replaced
- 110
does javascript have dictionaries
- 111
does javascript have garbage collection
- 112
will javascript be replaced reddit
- 113
is javascript a programming language
- 114
is javascript outdated
- 115
how javascript works under the hood
- 116
which javascript framework should i learn
- 117
which javascript framework is best
- 118
do i have javascript enabled
- 119
how would you call a function in javascript
- 120
how javascript async works
- 121
can javascript be multithreaded
- 122
who updates javascript
- 123
when javascript was invented month
- 124
what would you use object-oriented javascript for
- 125
who call function javascript
- 126
should javascript be on or off on iphone
- 127
how javascript sort works
- 128
can javascript be used for game development
- 129
does javascript need semicolons
- 130
why would javascript be disabled
- 131
who created javascript language
- 132
does hackerrank have javascript
- 133
is javascript object oriented
- 134
who created javascript
- 135
does javascript have classes
- 136
have javascript error
- 137
should javascript be capitalized
- 138
do javascript arrays maintain order
- 139
when javascript was developed
- 140
are javascript sets ordered
- 141
did javascript come from java
- 142
was javascript built in 10 days
- 143
where javascript is used
- 144
why javascript for web development
- 145
can javascript access local files
- 146
should javascript be enabled on safari
- 147
should javascript be on or off
- 148
have javascript run on page load
- 149
why javascript over typescript
- 150
does ipad have javascript
- 151
can javascript read httponly cookie
- 152
does javascript have pointers
- 153
where javascript code is executed
- 154
should javascript be in a separate file
- 155
do javascript have classes
- 156
when javascript function
- 157
which javascript framework is best for backend
- 158
does chromebook have javascript
- 159
can javascript be used to make games
- 160
how javascript works
- 161
who owns javascript
- 162
was javascript written in 10 days
More Sitesto check out once you're finished browsing here.
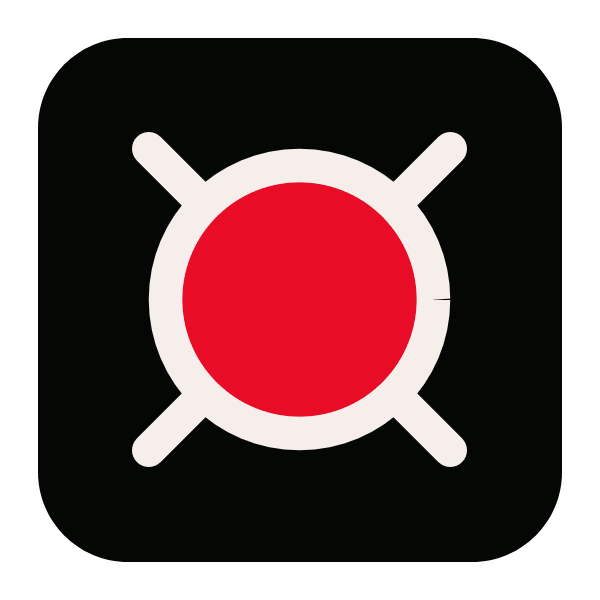
https://www.0x3d.site/
0x3d is designed for aggregating information.
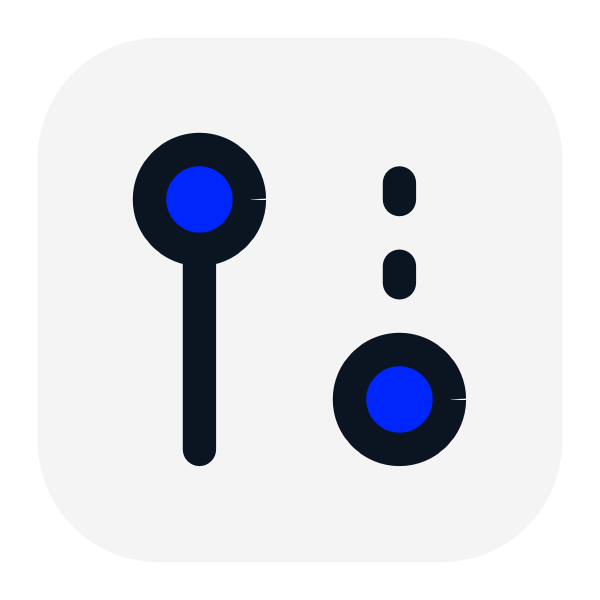
https://nodejs.0x3d.site/
NodeJS Online Directory

https://cross-platform.0x3d.site/
Cross Platform Online Directory
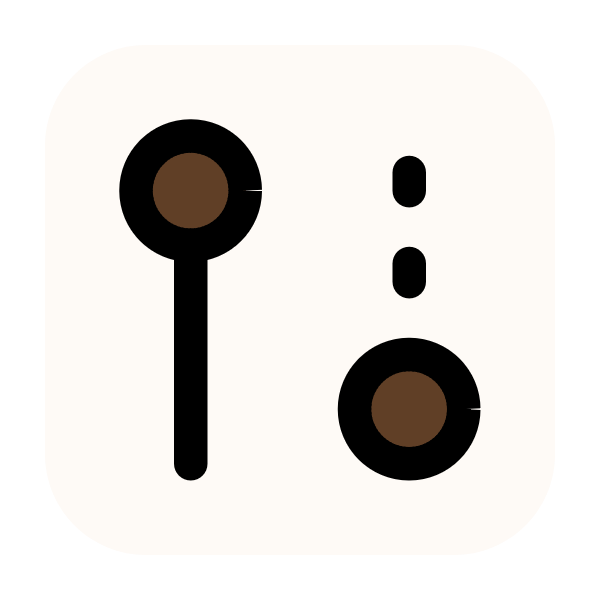
https://open-source.0x3d.site/
Open Source Online Directory
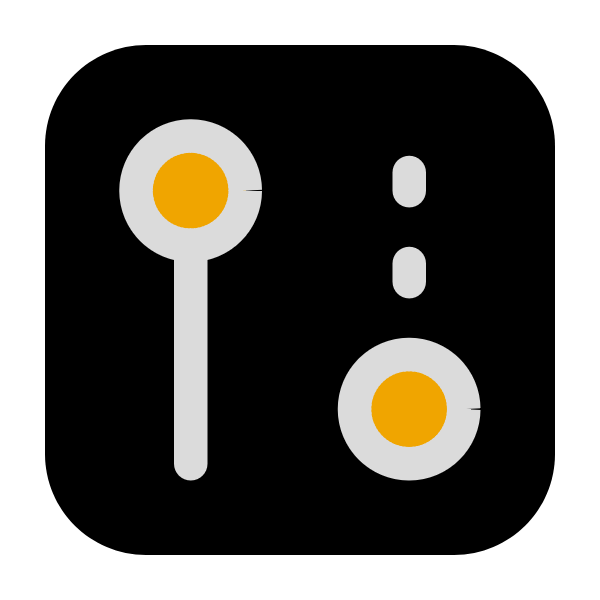
https://analytics.0x3d.site/
Analytics Online Directory
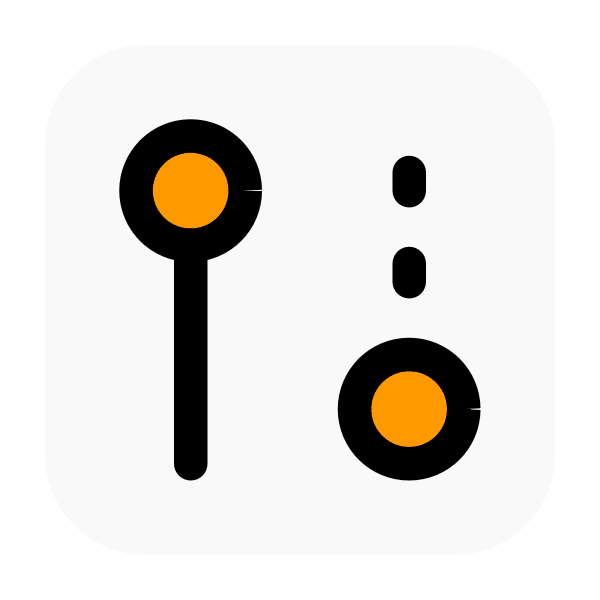
https://javascript.0x3d.site/
JavaScript Online Directory

https://golang.0x3d.site/
GoLang Online Directory
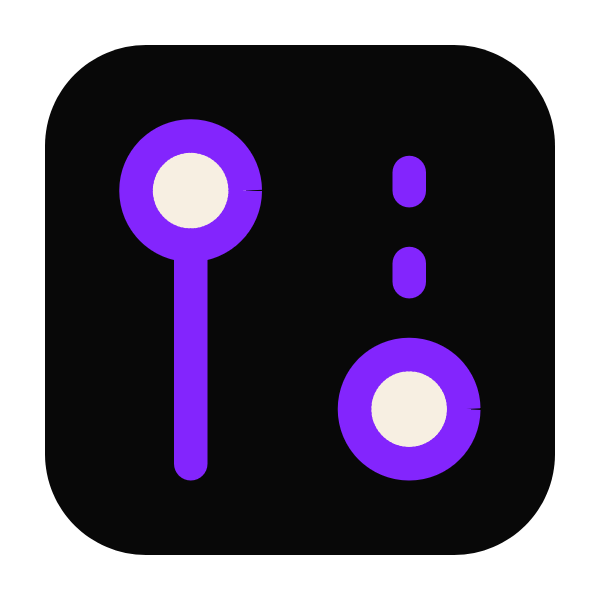
https://python.0x3d.site/
Python Online Directory

https://swift.0x3d.site/
Swift Online Directory

https://rust.0x3d.site/
Rust Online Directory
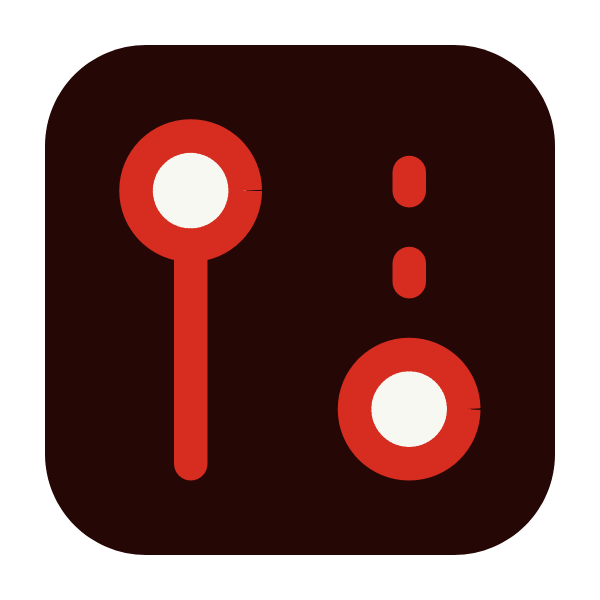
https://scala.0x3d.site/
Scala Online Directory

https://ruby.0x3d.site/
Ruby Online Directory

https://clojure.0x3d.site/
Clojure Online Directory
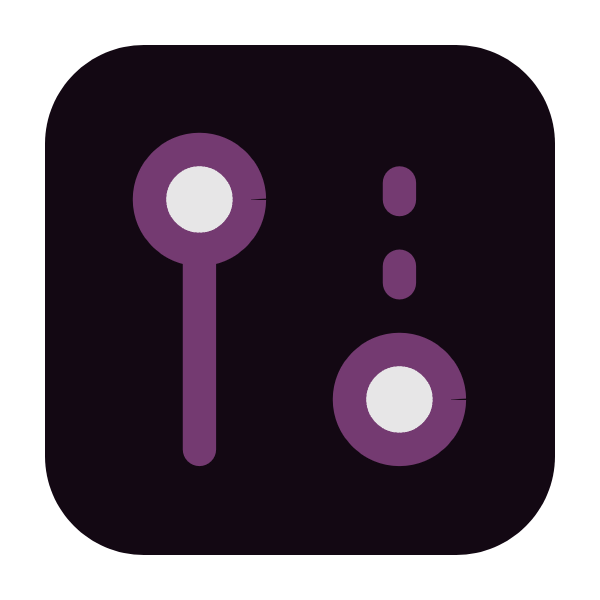
https://elixir.0x3d.site/
Elixir Online Directory
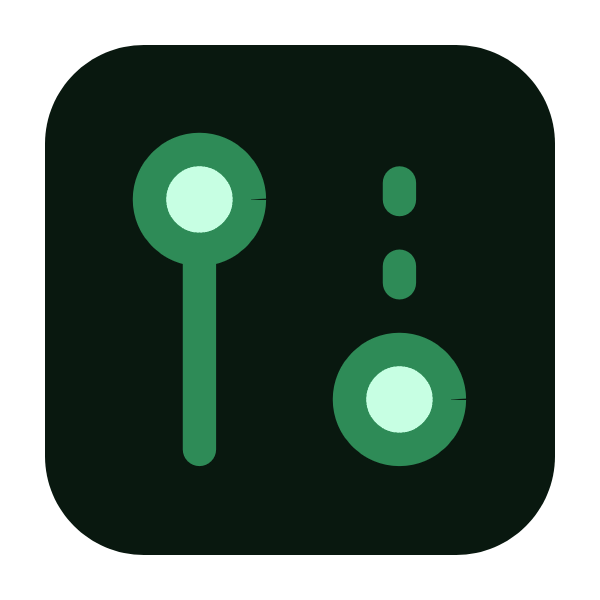
https://elm.0x3d.site/
Elm Online Directory

https://lua.0x3d.site/
Lua Online Directory
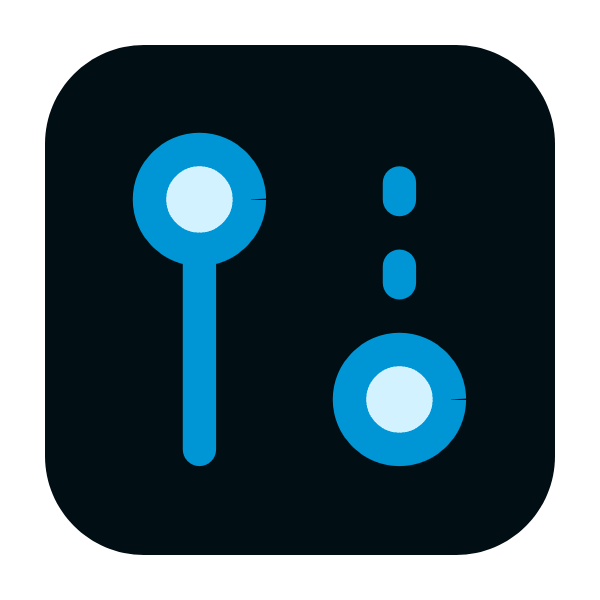
https://c-programming.0x3d.site/
C Programming Online Directory
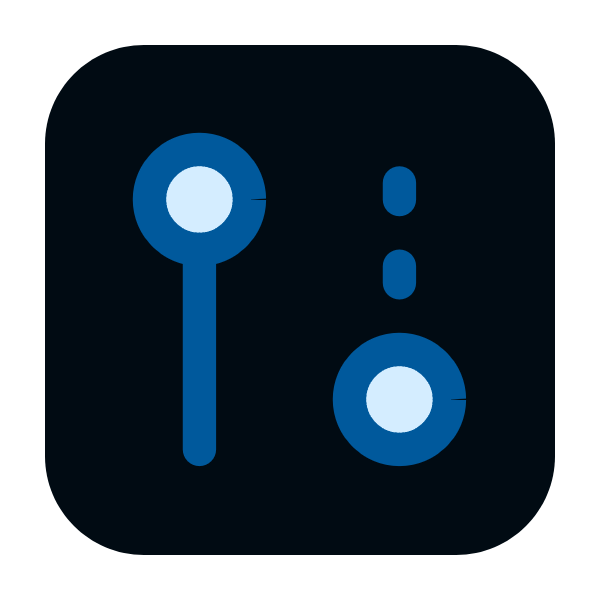
https://cpp-programming.0x3d.site/
C++ Programming Online Directory

https://r-programming.0x3d.site/
R Programming Online Directory

https://perl.0x3d.site/
Perl Online Directory
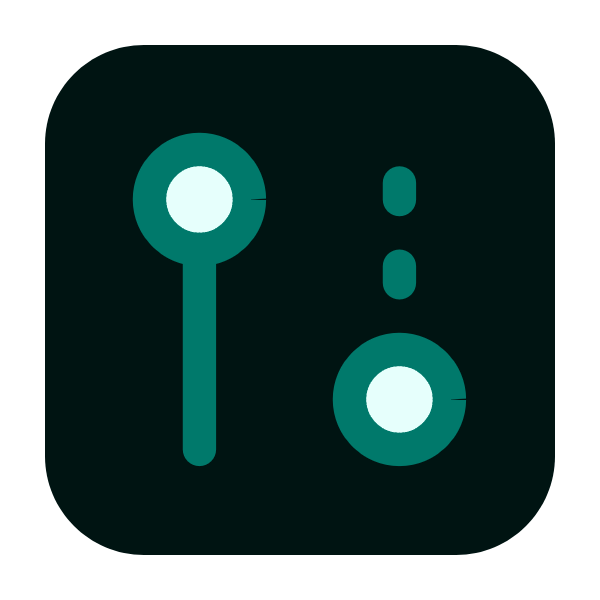
https://java.0x3d.site/
Java Online Directory

https://kotlin.0x3d.site/
Kotlin Online Directory

https://php.0x3d.site/
PHP Online Directory

https://react.0x3d.site/
React JS Online Directory
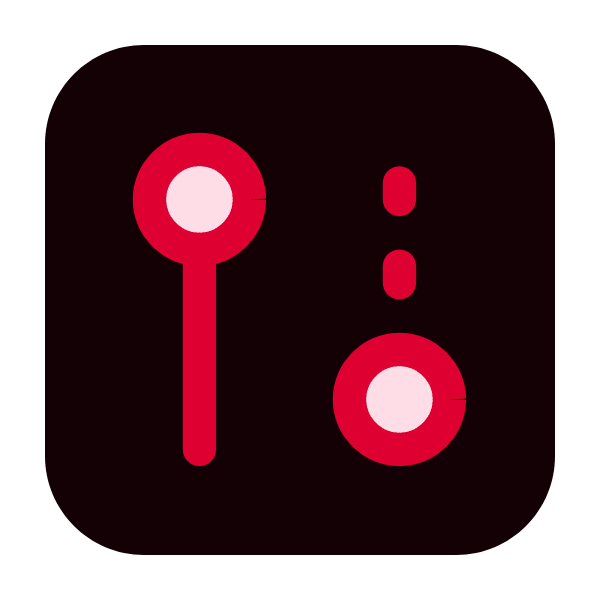
https://angular.0x3d.site/
Angular JS Online Directory